A dead man's switch is a fairly simple concept. If you don't perform a specific task before a set amount of time, it'll perform a specific action you set. They can be handy not just for hackers but for everyone who wants to protect themselves, someone else, or something tangible or intangible from harm. While there are more nefarious uses for a dead man's switch, white hats can put one to good use.
These switches have appeared in pop culture in many different forms, and examples can be seen in films such as "Point Break," "Speed," and "Crimson Tide." For a more recent example, in the film "Tenet," Sator (the antagonist) had implemented a dead man's switch to go off whenever his heart stopped, which would activate the algorithm that would end the entire world.
Notorious whistleblower Edward Snowden even used one in real life when he leaked all of those sensitive NSA documents to journalists. He sent encrypted versions to people he could trust who would receive the decryption key if he were to die under mysterious or violent circumstances.
When a Dead Man's Switch Comes in Handy
From a white hat's perspective, or just someone wanting to protect themselves from data theft by black hats or law enforcement, a dead man's switch is most useful for locking things down. Let's say you're in a protest with the possibility of getting arrested. You could set a kill switch for your laptop in case you don't check in via Twitter every so often. That way, it's harder for anyone to access your data.
Another scenario involves just wanting to keep some sensitive files locked down before anyone has a chance to access them. If you don't perform a specific action that you set for yourself, either once or in intervals, then the files you selected would be encrypted so that nobody could read them.
Building a Simple Dead Man's Witch in Python 3
In this guide, we'll be using Python 3 to write a simple dead man's switch that does just that — encrypts a file if we don't check in when specified. Now we could make one that sends a password out for an already encrypted file if we don't check in when specified, but then you have to deal with Twitter APIs, and that's a little bit more complicated. We just want to get you started so you can branch out to more advanced dead man's switches afterward.
Python 3 and a Python 3 integrated development environment (IDE) is all you really need to build one. We'll be using PyCharm as our IDE. To follow along, here's the full Python code that we've made, but skip below to find out how it works.
import twint, time, datetime, pyAesCrypt
# This is the payload - It will encrypt whatever file we specified. I commented out the part that deletes the original file
def FirePayload(filePath, encryptPass):
print("ACTIVATED PAYLOAD!!!!!")
bufferSize = 64 * 1024 # encryption/decryption buffer size - 64K
pyAesCrypt.encryptFile(filePath, (filePath+'.aes'), encryptPass, bufferSize) # encrypt
# pyAesCrypt.decryptFile("data.txt.aes", "dataout.txt", password, bufferSize) decrypt
# secure_delete.secure_random_seed_init(); secure_delete.secure_delete('/Users/skickar/Desktop/data.txt') # Erases the plaintext file
print("SWITCH ACTIVATED - LOCKDOWN MODE ENTERED")
exit()
# Checks for the keyphrase on Twitter, checks if the time is up
def CheckKey(c, delayTime, filePath, encryptPass, targetTime):
try:
twint.run.Search(c)
except ValueError:
print("Something bad happen")
GetTargets()
tweets = twint.output.tweets_list
if not tweets:
if (time.time() >= targetTime): FirePayload(filePath, encryptPass)
else:
print("No results, trying again after delay")
time.sleep(delayTime)
CheckKey(c, delayTime, filePath, encryptPass, targetTime)
else:
print("Deadswitch De-Activated, Entered Safe Mode")
exit()
# Gets the information to run the loop, such as the keyword, file to encrypt, and how long to run
def GetTargets():
c = twint.Config()
startTime = input("Date to start searching (format: %Y-%m-%d)\n>")
try: datetime.datetime.strptime(startTime, '%Y-%m-%d')
except ValueError:
print("That's not a date, try again (format: %Y-%m-%d)")
GetTargets()
c.Since = startTime
c.Search = input("Keyphrase to disarm switch?\n>")
c.Username = input("Twitter account to watch?\n>")
delayTime = int(input("Time in seconds to wait between checking the account?\n>"))
filePath = input("File to encrypt if switch fires?\n>")
encryptPass = input("Password to encrypt file?\n>")
targetTime = (time.time() + (int(input("How many minutes to run before firing?\n>"))*60))
c.Hide_output = True
c.Store_object = True
CheckKey(c, delayTime, filePath, encryptPass, targetTime)
GetTargets()
Download the Python Code
While the full Python code is visible in the code box above, you can check it out on GitHub or download it to your computer with the following command.
~$ git clone https://github.com/skickar/DeadManSwitch.git
Cloning into 'DeadManSwitch'...
remote: Enumerating objects: 3, done.
remote: Counting objects: 100% (3/3), done.
remote: Compressing objects: 100% (2/2), done.
remote: Total 3 (delta 0), reused 0 (delta 0), pack-reused 0
Unpacking objects: 100% (3/3), 1.52 KiB | 1.52 MiB/s, done.
Install the Required Libraries
Our Python code has the time and datetime libraries, which are standard libraries that should already be on your system. With these, the code can deal with timing matters and the parsing of date/time formats. But there are a few other libraries that you'll need as well. Specifically, Twint and PyAEScrypt.
Twint is a Twitter scraping tool that helps you grab a user's recent tweets, locate specific tweets for historical data, export evidence and metadata, collect real-time data, and more. In our project today, we'll use Twint as the mechanism for our dead man's switch.
PyAEScrypt is a tool that lets you encrypt and decrypt a file quickly. In our case, that's our payload, where we'll be encrypting a file with a password in the event that we don't check-in. Use pip3 install twint pyAesCrypt to install both dependencies.
~$ cd /home/kali/DeadManSwitch
~/DeadManSwitch$ pip3 install twint pyAesCrypt
Defaulting to user installation because normal site-packages is not writeable
Collecting twint
Downloading twint-2.1.20.tar.gz (31 kB)
Collecting pyAesCrypt
Downloading pyAesCrypt-0.4.3-py3-none-any.whl (15 kB)
Requirement already satisfied: aiodns in /usr/lib/python3/dist-packages (from twint) (2.0.0)
Requirement already satisfied: aiohttp in /usr/lib/python3/dist-packages (from twint) (3.6.2)
Collecting aiohttp_socks
Downloading aiohttp_socks-0.5.5-py3-none-any.whl (9.1 kB)
Requirement already satisfied: beautifulsoup4 in /usr/lib/python3/dist-packages (from twint) (4.9.1)
Collecting cchardet
Downloading cchardet-2.1.7-cp38-cp38-manylinux2010_x86_64.whl (265 kB)
|████████████████████████████████| 265 kB 2.1 MB/s
Collecting elasticsearch
Downloading elasticsearch-7.9.1-py2.py3-none-any.whl (219 kB)
|████████████████████████████████| 219 kB 2.1 MB/s
Collecting fake-useragent
Downloading fake-useragent-0.1.11.tar.gz (13 kB)
Collecting geopy
Downloading geopy-2.0.0-py3-none-any.whl (111 kB)
|████████████████████████████████| 111 kB 3.0 MB/s
Collecting googletransx
Downloading googletransx-2.4.2.tar.gz (13 kB)
Requirement already satisfied: pandas in /usr/lib/python3/dist-packages (from twint) (0.25.3)
Requirement already satisfied: pysocks in /usr/lib/python3/dist-packages (from twint) (1.6.8)
Collecting schedule
Downloading schedule-0.6.0-py2.py3-none-any.whl (8.7 kB)
Requirement already satisfied: cryptography in /usr/lib/python3/dist-packages (from pyAesCrypt) (2.8)
Collecting python-socks[asyncio]>=1.0.1
Downloading python_socks-1.1.0-py3-none-any.whl (32 kB)
Requirement already satisfied: attrs>=19.2.0 in /usr/lib/python3/dist-packages (from aiohttp_socks->twint) (19.3.0)
Requirement already satisfied: certifi in /usr/lib/python3/dist-packages (from elasticsearch->twint) (2020.4.5.1)
Requirement already satisfied: urllib3>=1.21.1 in /usr/lib/python3/dist-packages (from elasticsearch->twint) (1.25.9)
Collecting geographiclib<2,>=1.49
Downloading geographiclib-1.50-py3-none-any.whl (38 kB)
Requirement already satisfied: requests in /usr/lib/python3/dist-packages (from googletransx->twint) (2.23.0)
Requirement already satisfied: async-timeout>=3.0.1; extra == "asyncio" in /usr/lib/python3/dist-packages (from python-socks[asyncio]>=1.0.1->aiohttp_socks->twint) (3.0.1)
Building wheels for collected packages: twint, fake-useragent, googletransx
Building wheel for twint (setup.py) ... done
Created wheel for twint: filename=twint-2.1.20-py3-none-any.whl size=33919 sha256=d25c8ecc0e6d1f500a7826f67e04112e74c2b73c3c19b46e912e7082701fb8c6
Stored in directory: /home/kali/.cache/pip/wheels/4e/80/ea/5b82b5140b7dec857ec0bbfe908193ebf2e5d7f2ebee297519
Building wheel for fake-useragent (setup.py) ... done
Created wheel for fake-useragent: filename=fake_useragent-0.1.11-py3-none-any.whl size=13487 sha256=fbe421108a3a3f10e2b6152ea2aeaf48723cdf98a488084e16d2409d252edd5f
Stored in directory: /home/kali/.cache/pip/wheels/a0/b8/b7/8c942b2c5be5158b874a88195116b05ad124bac795f6665e65
Building wheel for googletransx (setup.py) ... done
Created wheel for googletransx: filename=googletransx-2.4.2-py3-none-any.whl size=15971 sha256=c9f6b5d407918e73dd265146f2a10669820d0f110183dd157a9e40b509fb4921
Stored in directory: /home/kali/.cache/pip/wheels/6a/54/98/96b62f08dd73eca3147e36b5099d77c3f8fedc5472bb488167
Successfully built twint fake-useragent googletransx
Installing collected packages: python-socks, aiohttp-socks, cchardet, elasticsearch, fake-useragent, geographiclib, geopy, googletransx, schedule, twint, pyAesCrypt
WARNING: The script twint is installed in '/home/kali/.local/bin' which is not on PATH.
Consider adding this directory to PATH or, if you prefer to suppress this warning, use --no-warn-script-location.
Successfully installed aiohttp-socks-0.5.5 cchardet-2.1.7 elasticsearch-7.9.1 fake-useragent-0.1.11 geographiclib-1.50 geopy-2.0.0 googletransx-2.4.2 pyAesCrypt-0.4.3 python-socks-1.1.0 schedule-0.6.0 twint-2.1.20
WARNING: You are using pip version 20.2.2; however, version 20.2.4 is available.
You should consider upgrading via the '/usr/bin/python3 -m pip install --upgrade pip' command.
If you're using PyCharm, cd to the folder where you downloaded and extracted the PyCharm program, go into that bin folder, and use ./pycharm.sh to open up the PyCharm IDE. Then, create a new project, labeled something like "DeadManSwitch."
~/DeadManSwitch$ cd /home/kali/pycharm-community-2020.2.3/bin
~/pycharm-community-2020.2.3/bin$ ./pycharm.sh
Picked up _JAVA_OPTIONS: -Dawt.useSystemAAFontSettings=on -Dswing.aatext=true
OpenJDK 64-Bit Server VM warning: Option UseConcMarkSweepGC was deprecated in version 9.0 and will likely be removed in a future release.
Oct 28, 2020 4:02:00 PM java.util.prefs.FileSystemPreferences$1 run
INFO: Created user preferences directory.
Oct 28, 2020 4:02:00 PM java.util.prefs.FileSystemPreferences$6 run
WARNING: Prefs file removed in background /home/kali/.java/.userPrefs/prefs.xml
2020-10-28 16:02:12,943 [ 12894] WARN - llij.ide.plugins.PluginManager - Resource bundle redefinition for plugin 'com.jetbrains.pycharm.community.customization'. Old value: messages.ActionsBundle, new value: messages.PyBundle
2020-10-28 16:02:14,581 [ 14532] WARN - llij.ide.plugins.PluginManager - Resource bundle redefinition for plugin 'com.jetbrains.pycharm.community.customization'. Old value: messages.ActionsBundle, new value: messages.PyBundle
You could also add Twint and pyAesCrypt manually in the PyCharm IDE if you don't want to deal with pip or when you want to be sure that you have everything you need. If you want to do that, select "File," then "Settings," and choose your dead man's switch project. Next, select "Python Interpreter," and click the plus (+) sign to search for and add "twint" and "pyAesCrypt" packages.
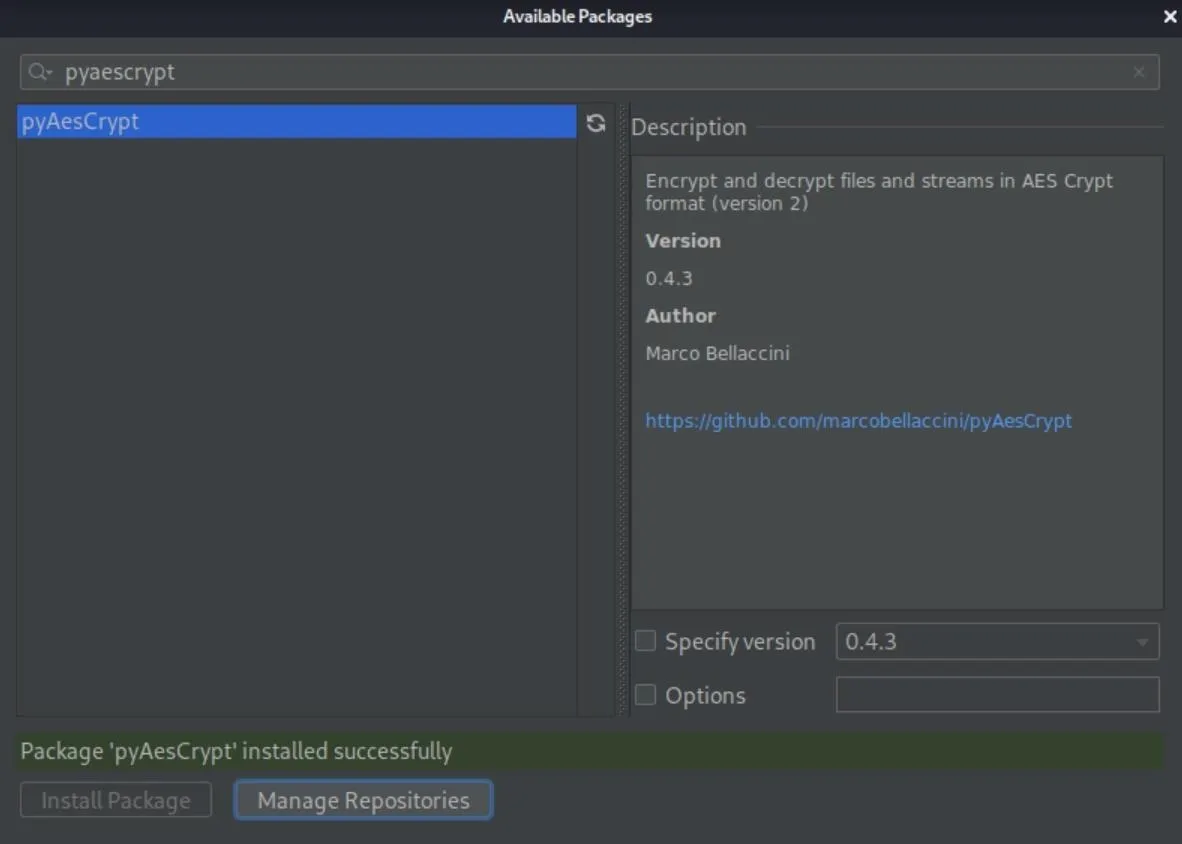
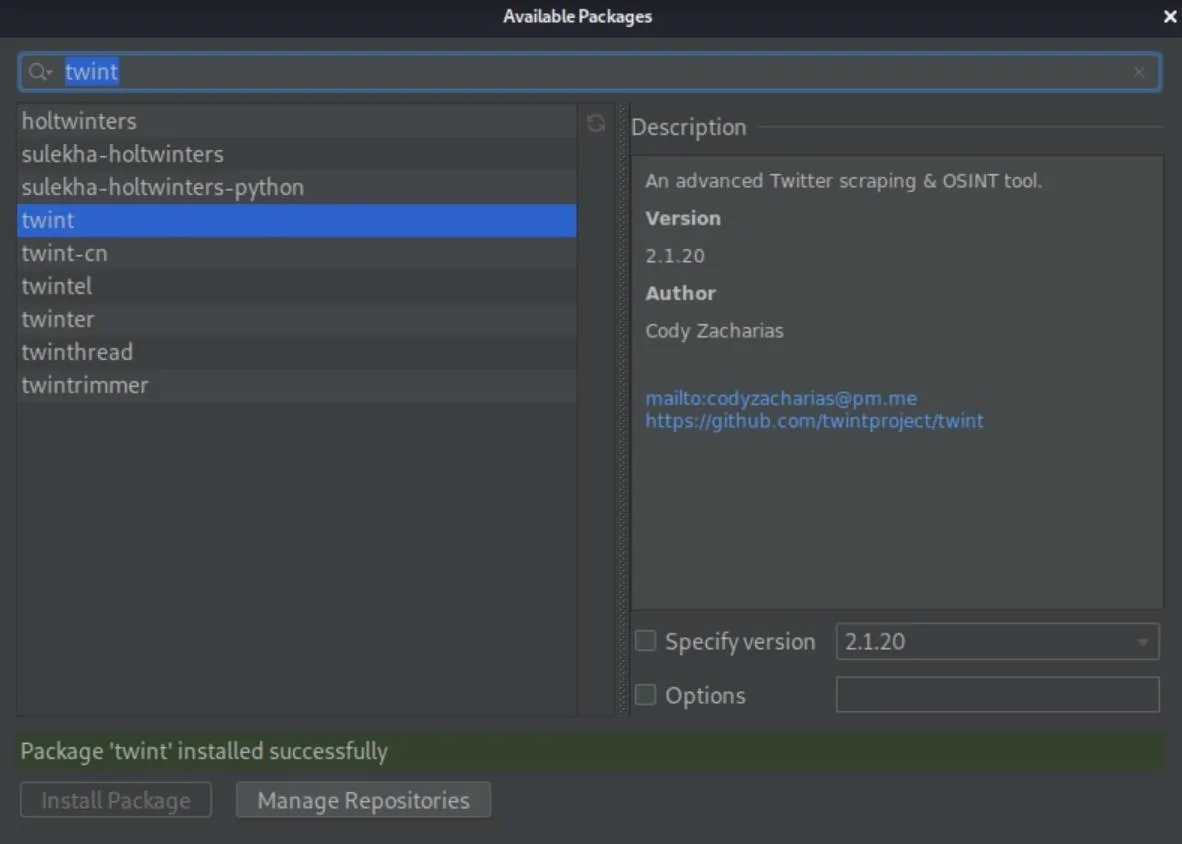
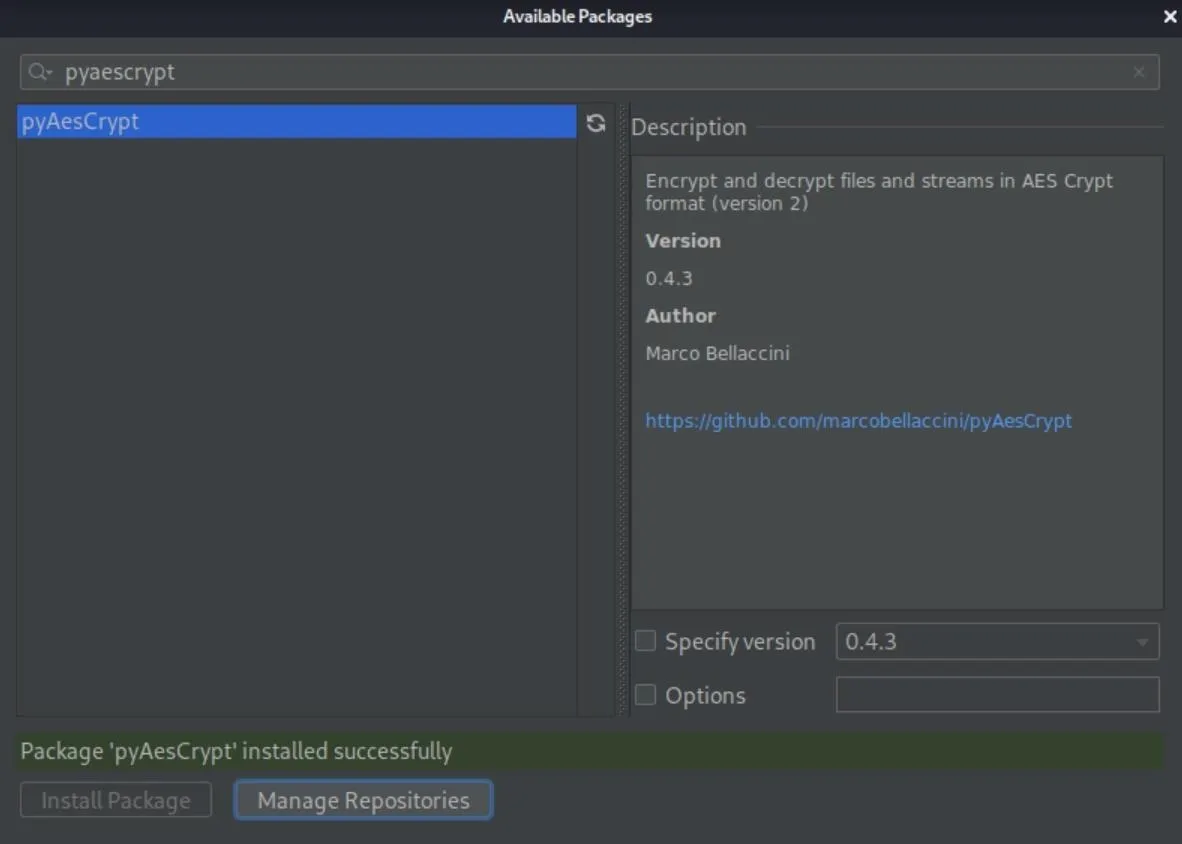
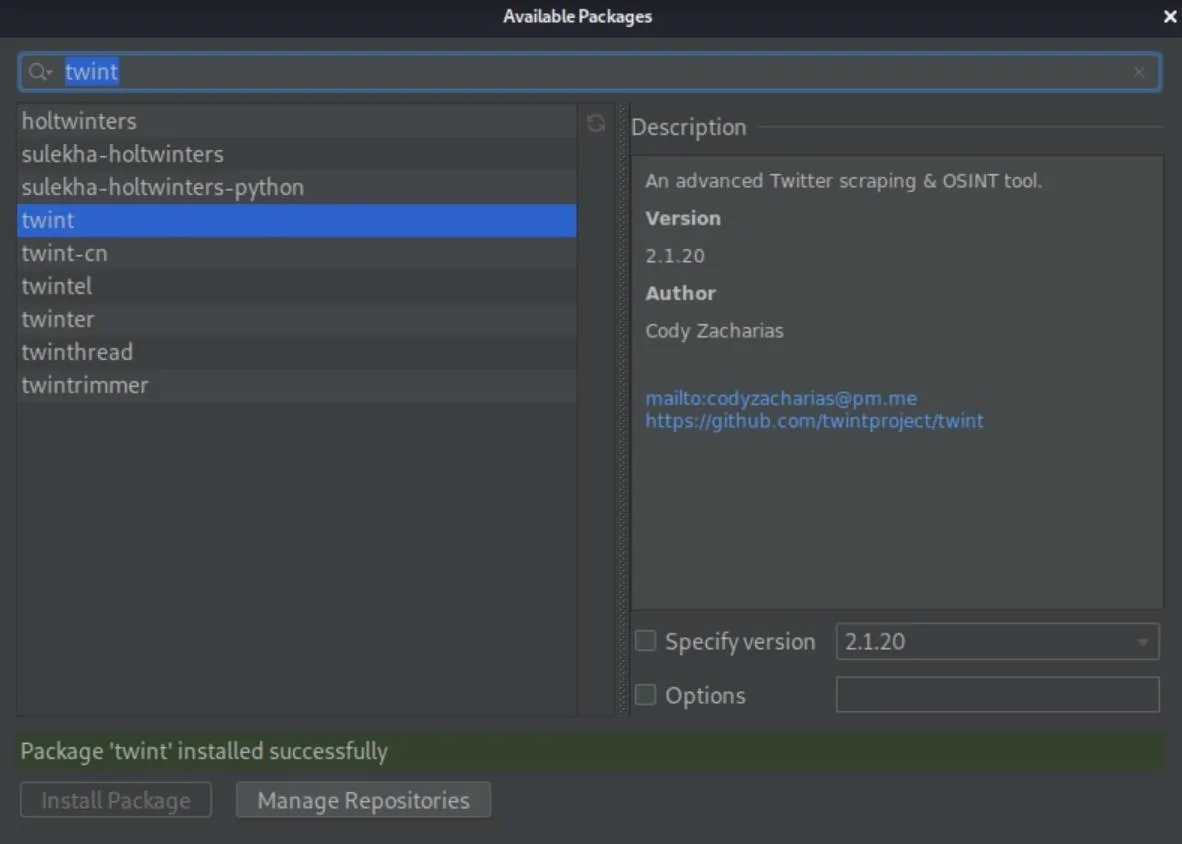
Build the Code
Now we're ready to start writing the code. We want to make sure that we can, over a certain period of time, either check-in or not check-in. And based on that exchange, the file we choose will be encrypted. There are three main functions of this project. Let's first focus on the targeting function.
The Targeting Function
This is the section of the code that gets all of the information to make our project run. The last GetTargets() is a single function that calls all of the other functions.
For the targeting aspect, we start with def GetTargets():, and then we create a Twint object using c = twint.Config(). This is where you would start to include all of the configuration items you want to run a search on, such as a username, the date and time that you want to start the search, etc.
# Gets the information to run the loop, such as the keyword, file to encrypt, and how long to run
def GetTargets():
c = twint.Config()
For the startTime, we want to add in the current date, or the date we want Twint to exclude everything that came before it so that we're not getting any false positives from code phrases that appear in the timeline elsewhere. The input part will appear on the screen when the program runs, and the user of the program will need to enter the date in the correct format specified.
Since the date format is particular, we have some code to let us know if the user is using the wrong date format since Twint is picky. If it is wrong, it'll print the message "That's not a date, try again." Then, it'll bounce back to the start to ask for the date again.
# Gets the information to run the loop, such as the keyword, file to encrypt, and how long to run
def GetTargets():
c = twint.Config()
startTime = input("Date to start searching (format: %Y-%m-%d)\n>")
try: datetime.datetime.strptime(startTime, '%Y-%m-%d')
except ValueError:
print("That's not a date, try again (format: %Y-%m-%d)")
GetTargets() ##Making sure the format is correct for the data
Next, we recall the startTime we just went over with c.Since, which is the first moving piece of c, the Twint configuration. Then we need to ask the user for the search term with c.Search, which could be a word or phrase the user wants to look out for. It will ask, "Keyphrase to disarm switch?"
After that, we use c.Username to ask the user which Twitter account it should be watching. Specifically, it will ask the user, "Twitter account to watch?"
Next, with delayTime, we say how often the program should check the Twitter user's timeline for that keyword or phrase; it's in seconds, but you could make it as long or short as you want. What the user will see in the program is "Time in seconds to wait between checking the account?"
# Gets the information to run the loop, such as the keyword, file to encrypt, and how long to run
def GetTargets():
c = twint.Config()
startTime = input("Date to start searching (format: %Y-%m-%d)\n>")
try: datetime.datetime.strptime(startTime, '%Y-%m-%d')
except ValueError:
print("That's not a date, try again (format: %Y-%m-%d)")
GetTargets() ##Making sure the format is correct for the data
c.Since = startTime
c.Search = input("Keyphrase to disarm switch?\n>")
c.Username = input("Twitter account to watch?\n>")
delayTime = int(input("Time in seconds to wait between checking the account?\n>"))
For the filePath line, we ask the user running our program, "File to encrypt if switch fires?" That's where the file path goes for the file that will be encrypted should the keyword or phrase not be detected over the set target time.
The encryptPass line, asking "Password to encrypt file?" will encrypt the file with the password the user chooses. Then, the line that starts with targetTime asks "How many minutes to run before firing?" This is the target time between when the time elapsed without the safety word/phrase and when the encryption should happen.
# Gets the information to run the loop, such as the keyword, file to encrypt, and how long to run
def GetTargets():
c = twint.Config()
startTime = input("Date to start searching (format: %Y-%m-%d)\n>")
try: datetime.datetime.strptime(startTime, '%Y-%m-%d')
except ValueError:
print("That's not a date, try again (format: %Y-%m-%d)")
GetTargets() ##Making sure the format is correct for the data
c.Since = startTime
c.Search = input("Keyphrase to disarm switch?\n>")
c.Username = input("Twitter account to watch?\n>")
delayTime = int(input("Time in seconds to wait between checking the account?\n>"))
filePath = input("File to encrypt if switch fires?\n>")
encryptPass = input("Password to encrypt file?\n>")
targetTime = (time.time() + (int(input("How many minutes to run before firing?\n>"))*60))
Now we need to tell Twint how we want to store the file. The c.Hide_output line says that we want to hide the output so that it only shows up in the list we designate later, and c.Store_object says we want to store the info, which correlates with tweets = twint.output.tweets_list in the checking function.
In the last line, we call all of the key details over to the checking function called CheckKey, which will continuously check for our targeting information, including everything in c, the Twint configuration, as well as delayTime, filePath, encryptPass, and targetTime.
# Gets the information to run the loop, such as the keyword, file to encrypt, and how long to run
def GetTargets():
c = twint.Config()
startTime = input("Date to start searching (format: %Y-%m-%d)\n>")
try: datetime.datetime.strptime(startTime, '%Y-%m-%d')
except ValueError:
print("That's not a date, try again (format: %Y-%m-%d)")
GetTargets() ##Making sure the format is correct for the data
c.Since = startTime
c.Search = input("Keyphrase to disarm switch?\n>")
c.Username = input("Twitter account to watch?\n>")
delayTime = int(input("Time in seconds to wait between checking the account?\n>"))
filePath = input("File to encrypt if switch fires?\n>")
encryptPass = input("Password to encrypt file?\n>")
targetTime = (time.time() + (int(input("How many minutes to run before firing?\n>"))*60))
c.Hide_output = True
c.Store_object = True
CheckKey(c, delayTime, filePath, encryptPass, targetTime)
GetTargets()
The Checking Function
The checking function is the part that actually performs the search on Twint. The first def line tasks the information from the targeting function that we specified, including c, delayTime, filePath, encryptPass, and targetTime.
Then, if something goes wrong, like the date is wrong, we can print an error message for ValueError so that we know something happened, and then it will return to GetTargets() in the targeting function to start again.
# Checks for the keyphrase on Twitter, checks if the time is up
def CheckKey(c, delayTime, filePath, encryptPass, targetTime):
try:
twint.run.Search(c)
except ValueError:
print("Something bad happen")
GetTargets()
Next, we create a list called "tweets" with twint.output.tweets_list so that all of the tweets found that match the target word or phrase can be dumped in it. The whole program will be watching this list, so it's a pretty big part of the code.
# Checks for the keyphrase on Twitter, checks if the time is up
def CheckKey(c, delayTime, filePath, encryptPass, targetTime):
try:
twint.run.Search(c)
except ValueError:
print("Something bad happen")
GetTargets()
tweets = twint.output.tweets_list
The next line will check to see if there is anything in the tweets list. If there is something in there, we know that the person who ran this program checked in, and everything is fine.
If it detects no tweets in the list, we compare the current time using time.time(), noting if it's greater than or equal to (>=) the targetTime. If it is, then it will fire the payload function, pointing to the file path and encryption password.
# Checks for the keyphrase on Twitter, checks if the time is up
def CheckKey(c, delayTime, filePath, encryptPass, targetTime):
try:
twint.run.Search(c)
except ValueError:
print("Something bad happen")
GetTargets()
tweets = twint.output.tweets_list
if not tweets:
if (time.time() >= targetTime): FirePayload(filePath, encryptPass)
The else statement for the delayTime is for how often it should check the user's Twitter timeline. This will be the delayTime from the targeting function, and it will then start back at the beginning of the checking function to try again.
# Checks for the keyphrase on Twitter, checks if the time is up
def CheckKey(c, delayTime, filePath, encryptPass, targetTime):
try:
twint.run.Search(c)
except ValueError:
print("Something bad happen")
GetTargets()
tweets = twint.output.tweets_list
if not tweets:
if (time.time() >= targetTime): FirePayload(filePath, encryptPass)
else:
print("No results, trying again after delay")
time.sleep(delayTime)
CheckKey(c, delayTime, filePath, encryptPass, targetTime)
If there is something in the list, we have an else statement to print a message saying that the dead man's switch has been deactivated and is in safe mode. If that's the case, the program will then complete, and the file is in no need of being encrypted.
# Checks for the keyphrase on Twitter, checks if the time is up
def CheckKey(c, delayTime, filePath, encryptPass, targetTime):
try:
twint.run.Search(c)
except ValueError:
print("Something bad happen")
GetTargets()
tweets = twint.output.tweets_list
if not tweets:
if (time.time() >= targetTime): FirePayload(filePath, encryptPass)
else:
print("No results, trying again after delay")
time.sleep(delayTime)
CheckKey(c, delayTime, filePath, encryptPass, targetTime)
else:
print("Deadswitch De-Activated, Entered Safe Mode")
exit()
The Payload Function
Now for the last remaining part of the puzzle: the payload. If the keyword or phrase had not been detected in the checking function after a set amount of time, it would call the payload here. And this is where pyAesCrypt comes into action. We print a message saying that it's activated, then encrypt the file using AES with the specified buffer size.
import twint, time, datetime, pyAesCrypt
# This is the payload - It will encrypt whatever file we specified. I commented out the part that deletes the original file
def FirePayload(filePath, encryptPass):
print("ACTIVATED PAYLOAD!!!!!")
bufferSize = 64 * 1024 # encryption/decryption buffer size - 64K
pyAesCrypt.encryptFile(filePath, (filePath+'.aes'), encryptPass, bufferSize) # encrypt
Now, the next two lines are commented out, but they're there in case you want to decrypt the file (pyAesCrypt.decryptFile) or delete the file (secure_delete). So if you wanted to decrypt a file instead, you could use that line instead of the pyAesCrypt.encryptFile one. But if you wanted to encrypt the file and delete it afterward, you can use the secure_delete module, which you will have to install as we did Twint and pyAesCrypt.
import twint, time, datetime, pyAesCrypt
# This is the payload - It will encrypt whatever file we specified. I commented out the part that deletes the original file
def FirePayload(filePath, encryptPass):
print("ACTIVATED PAYLOAD!!!!!")
bufferSize = 64 * 1024 # encryption/decryption buffer size - 64K
pyAesCrypt.encryptFile(filePath, (filePath+'.aes'), encryptPass, bufferSize) # encrypt
# pyAesCrypt.decryptFile("data.txt.aes", "dataout.txt", password, bufferSize) decrypt
# secure_delete.secure_random_seed_init(); secure_delete.secure_delete('/Users/skickar/Desktop/data.txt') # Erases the plaintext file
print("SWITCH ACTIVATED - LOCKDOWN MODE ENTERED")
exit()
Run the Code
Now, run the code in PyCharm, which you can do by clicking the play button. If it works, you should see the question asking for the date to start searching.
/home/kali/PycharmProjects/DeadManSwitch/venv/bin/python /home/kali/PycharmProjects/DeadManSwitch/main.py
Date to start searching (format: %Y-%m-%d)
>
After you input the date to start searching, it'll ask you for the key phrase to disarm the dead man's switch.
>2020-10-28
Keyphrase to disarm switch?
>
After adding the search term, specify the Twitter account to search.
>kali
Twitter account to watch?
>
Now, when you give it a username, it'll ask you the time in seconds to wait between checking the account. Remember, it's in seconds only.
>NullByte
Time in seconds to wait between checking the account?
>
Then, it will ask which file you want to encrypt should it not detect the search term in the time specified.
>10
File to encrypt if switch fires?
>
After that, enter in a good password for the file.
>/home/kali/Documents/importantstuff.txt
Password to encrypt file?
>
And then choose how many minutes you want the program to run before firing.
>chooseagoodpassword
How many minutes to run before firing?
>
In our example, if it didn't detect the key search term after 10 seconds, it'll try again until either the term has been identified or time runs out. In the latter case, the file will be encrypted.
>1
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
No results, trying again after delay
No results, trying again after delay
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
No results, trying again after delay
No results, trying again after delay
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
PAYLOAD ACTIVATED!!!!!
SWITCH ACTIVATED - LOCKDOWN MODE ENTERED
In our example, we only encrypted a file, so there will be a new encrypted version of the file in the same folder as the regular file. If we wanted to delete the original file while only keeping the encrypted one, that's when we would un-comment the secure_delete linen in the payload function.
If it were to detect the keyword or phrase in the allotted timeframe, you would see something like this instead:
>1
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
No results, trying again after delay
No results, trying again after delay
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
No results, trying again after delay
No results, trying again after delay
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
CRITICAL:root:twint.run:Twint:Feed:noDataExpecting value: line 1 column 1 (char 0)
Deadswitch De-Activated, Entered Safe Mode
If you get an error code, there could be a chance that Twitter changed something on its end, and Twint hasn't been updated yet to reflect those changes. In that case, you could submit a request on the project's GitHub repository. It could also be that you used the wrong Twitter username or that the user has a private account.
Cover image by Pavel Ignatov/123RF; Screenshots by Retia/Null Byte
Comments
Be the first, drop a comment!