Welcome back Java programmers!
In this tutorial we'll being going over Variables/DataTypes and Type-casting
So What Are Java DataTypes?
Like in many other programming languages, you have variable types. Such as ints, doubles, chars, etc.
They are used to instantiate the kind of variable that something is.
In Java, we use them to tell Java "Hi, this is the type of number/letter/string_of_words that I want to use for this variable called X"
In Java, we have these primitive data types (when instantiated, they MUST be in lower case):
int = "int" variables are considered "whole numbers" in math. It is a 32-bit sized variable
double = "double" variables are considered "decimals" in math. It is a 64-bit variable
short = "short" variables are similar to "int" variables in that they are both whole numbers. It is a 16-bit variable
long = "long" variables are similar to "int" and "short"; they have an 'L' after their number. It is a 64-bit variable
float = "float" variables are similar to "doubles", they have an 'f' after their number. It is a 32-bit variable.
char = "char" variables are letters. It is a 16-bit variable. Unlike a String, they need single quotes around their value, else Java thinks it is a String. (' a ')
boolean = "boolean" variables are True/False statements. It's size is not defined (in Java, the booleans "true" and "false" must be in lower case, else Java will think that they are variable names)
String = Strings are sentences. Their size is undefined. The word "String" must have the "S" capitalized. Unlike a char, they need double quotes around their value ("Some words here")
------------------------------
Now That We Have This Down, We Can Begin Instantiating Variables
Fire up your trusty IDE or text editor and write these lines:
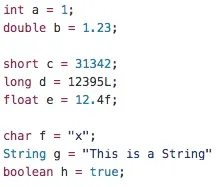
the grammar works this way:
[dataType] [nameOfTheVariable] = [value];
Spaces are needed between the bracketed words.
However they are not required between the [nameOfTheVariable] and the Equal (=) sign and the [value] part.
------------------------------
Now that we have variable instantiation down, lets look at Type Casting
What Is TypeCasting?
In short definition, it is to convert from one data type to another.
They can be used like this.
( Type of data type/primitive )name of variable of another data type;
For example, casting a double to an int will simply cut off the decimal point, and the numbers following it. So:
double x = 12.5;
int y = (int)x
will equal "12" because the ".5" is deleted.
Casting from an int to a double will simply add a .0 to the end of the int value. So:
int x = 5;
double y = (double)x;
will equal "5.0"
You can also cast a char into an int and vice versa:
int x = 65;
char y = (char)x"
Will equal the ASCII value of "65", which is: a capitalized "A"
You can all types, from one to another, EXCEPT Strings to/from Chars
---------------------------
I hope you enjoyed this tutorial,
Any questions, post in the comments
I apologize for not uploading a new tutorial last week (it has been busy for me)
-VoidX
Comments
Be the first, drop a comment!