Welcome back to another episode of Java Tutorials,
In today's tutorial, we'll be learning about what methods are, proper syntax when writing Methods, and why they are beneficial in a program's code.
Lets dive in.
--------------------------
What Is A Method
A method is essentially a "Group of code that together help in performing an operation.
There are 2 types of methods:
- The ones included in the JDK = These are the ones that Oracle (the Java creators) wrote. These include the functions such as println(), floor() (I'll teach about the Math functions in a later tutorial), and, but not limited to only these listed methods, toString()
- The ones you (the programmer) write = These will be the ones which are not included in the JDK, or the ones with the same name as one of the methods in the JDK, but you define as to what it does (using @Override , which will be explained in a later tutorial).
Why Are Methods Beneficial In Java
Short Answer: So you don't need to write the same code numerous amount of times to achieve one goal. It also makes your code look cleaner, (and for some programs) save space.
Method Syntax
Methods in Java are written in this way:
<Access type> <Static or not> <return type> <MethodName>(<a variable type and its name (optional)>){ }
Methods with a return type (that is not void) MUST return a variable of that same type. (otherwise Java complains and Eclipse raises errors)
So lets create a method that will determine whether a String variable has the word "hello" in that exact form
First, lets create a class:
(assuming you have already created a new Java file)
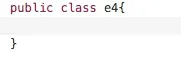
Now lets add our method.
For our purposes, we want the method to check whether the string contains "hello" and if it does, return true; if not, it returns false

Allow me to explain what I wrote:
- We wrote public because we want all methods within that class to be able to access the method.
- We wrote String because we wanted it to return a String that tells us whether String h contains the word "hello" or not
- We wrote containsHello because that is what we want to call our method (you can call your method anything you want, Java doesn't care (unless you name your method something that Java has in its JDK, then it raises errors.), just make sure you remember what that method does)
- We wrote (String h) inside of the parentheses because we want to be able to put a string into the method for when we call the method. Because we wrote String h, we have instantiated the variable "h" to be used in the method. If you try to call "h" outside of the method, Java will say that it does not exist, since it is defined only within our containsHello() method
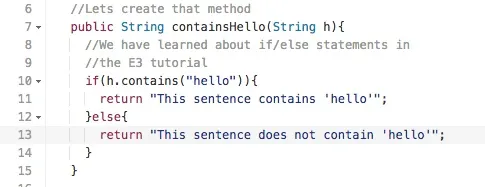
We have created an if/else to check for whether the string includes "hello"
The .contains("hello") part is simple. In the Java API
If it finds the charSequence (aka the word "hello") that we want to find, it returns true. If not, it returns false.
Now lets write our main method (Java programs can't run without a Main Method), and instantiate our sentence "String sentence" as we shall call it.

Since we learned about the main method back in E1, I won't go into explaining it.
However I will explain the two lines inside of the main method:
- We created a String called "sentence" and set it equal to "hello from the other side" (Don't ask why I wrote this. Adele was playing on the radio, this was the first sentence I thought of that contained the word "hello")
- Next we want our program to print what the answer is. So we write System.out.println(
- Next we call our containsHello(sentence) method and put our String sentence into the parentheses
(In Java, we call methods (of the same class) by directly writing their names and if it accepts a variable, we write it in the parentheses)
- Next, we close the print statement, add a semicolon ;
- Lastly, we make sure all parts of the code which require closing brackets are closed.
Now run the code, and we'll get the answer:
"This sentence does not contain 'hello'"
-------------------
As always, code is on GITHUB
Any questions/comments, feel free to post in the comments area
-VoidX
Comments
Be the first, drop a comment!