Welcome back, reader! In this tutorial, we will be covering our first program! So let's get to it.
We all know the unspoken tradition of the first program when learning a language and of course, here we will respect and complete it. Fire up your favorite text editor (be it vim, emacs, gedit, it's all the same to me, no h8) and try to keep up.
Example Code
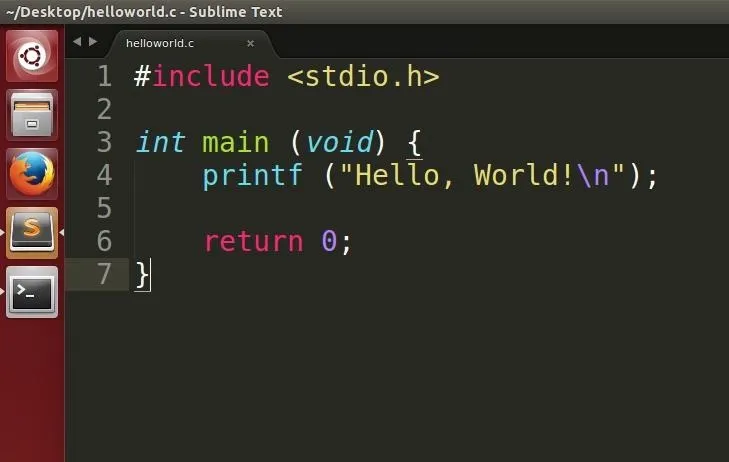
After writing this up, save it as helloworld.c (or whatever you wish with the .c extension, but keep in mind you will have to replace each instance of my file name with yours) and open up that terminal.
Note: Locate the directory of your C file and change your directory accordingly.
Compiling and Running
Again, for those who have IDEs, you may just press "Compile/Build and Run" and may skip the following step.
Here is the command we will be using:
- gcc helloworld.c
which will produce a file called a.out for those who are using any form of *nix. If you wish to specify an output file name, use gcc with the -o flag like so:
- gcc -o myprogram helloworld.c
which will produce an executable file called "myprogram" using the "helloworld.c" C file.
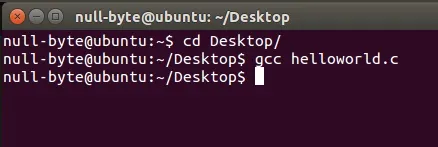
Now that we have successfully compiled our C file into an executable, let's run it. To run your executable file in the command line, simply type "./" followed by your executable file's name like so:
- ./a.out
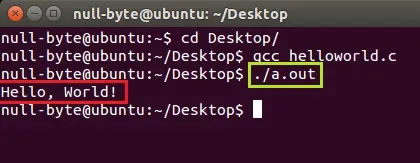
As you can see, the program has been successfully run and produces the output "Hello, World!" as we expected.
Code Analysis
In the first line we see #include <stdio.h>. This is a preprocessing directive used to include header files which contain the information of certain functions, in this case, printf is the function which is found in the stdio.h header file. Without this, the compiler will not be able to locate and build the function because it has not been defined so it does not know what it is. We will get into more detail on this in the future.
Next, we see int main (void). Main is absolutely required function for C programs because it is defined as the entry point of the program. Without the main function, the execution will not know where to start the program so if you try to build this without a function called main, the compiler will complain and give you an error. Following this, we see the open curly brace "{" which signifies the beginning of a function and at the end we can see the closing curly brace "}" which ends the defined function. But what does the int and void mean? Int is the return type of the function and void is the function's argument (or parameter), that is all I will say. We will cover this later when we learn functions. All programs we will be making in this tutorial will define main to return a type int because this is the standard and C programs are expected to return an int type.
The printf function is a function which allows us to print to the console. It will print whatever is inside the parenthesis with the double quotes. What's that "\n"? The \n is a special character which represents a newline character as you can see in the command line, we printed the text "Hello, World!" and then it printed out a new line. If this were not included, it would say "Hello, World!null-byte@ubuntu:~/Desktop$". We will go more into this later.
The return 0 is related to the return type of the function it is defined within. In this case, the return of 0 is related to the return type int of main and they must match otherwise it will not work and the compiler will moan and groan. What the return statement does is it returns execution control back to the calling function. The example we have is not enough to see very clearly but here, main's return gives control back to the operating system. Why 0? 0 means that execution of the program has been successful and that everything went as planned. Can you have any other numbers? Yes, of course, but the returned number usually identifies how the program terminated. All non-zero returns mean some form of abnormal termination where 1 is the most common, representing a failed execution of the program due to something not correctly working. Again, we will cover return statements in a tutorial on functions.
Conclusion
Phew! That was a quite a bit of content to soak in, if I might say so myself. Don't worry if it's all kind of confusing at the moment, everything will clear up as we go along but it certainly won't get any easier! Until next time.
dtm.
Comments
Be the first, drop a comment!