What's up guys! It's time to discuss strings in more detail.
Review
Just a revision in case you have forgotten about what buffers (arrays) are. A buffer is a container to hold data which are adjacent to each other in memory (we have seen this in the previous tutorial on memory). They have a set size which cannot be changed so it is important that you know how much space you need before declaring the array.
Strings
What is the definition of a string in C? A string is any combination of characters proceeded by a null terminating byte (\0). This means it can be a combination of ten characters, or one character, or even no characters! A string is defined with the combination of characters followed by a null terminator within a pair of double quotation marks, e.g. "This is a string".
Defining Strings
The following content will detail two common ways to define strings and what the situation and differences are per definition.
Character Array
We have seen the character array string definition before so here it is again in code:
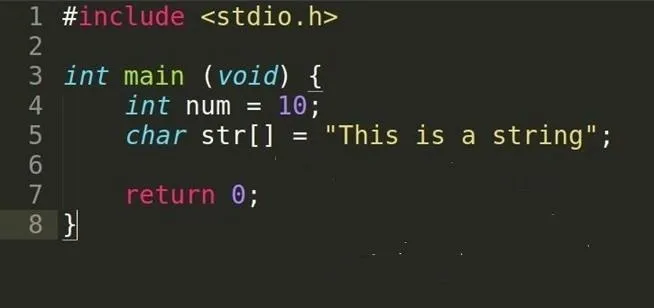
Just a reminder, the empty square brackets means that it will automatically complete the required number of elements needed to store the string including the null byte. You may also first declare the array with a predefined element number and then manually setting each element to a character.
Pointer to a Character
We have yet to talk about pointers however, for the sake of completeness, we will go over how to define a string with a character pointer.
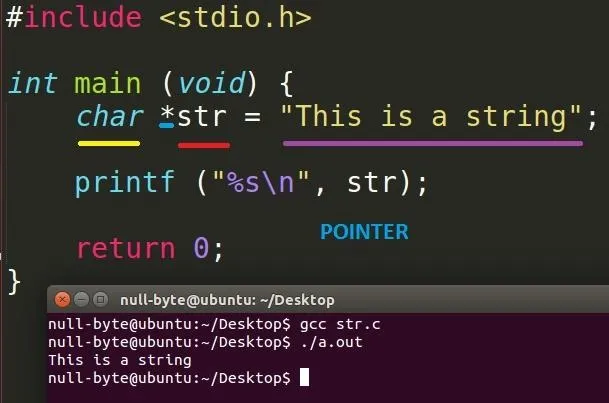
The variable "str" is declared as a "pointer to a character", so technically it is a pointer to the letter "T"of the string. However, when printing strings, remember that it will only stop printing wherever there is a null terminator therefore it still works. The difference between this and the character array is that the string content should not be modified. If you do so, it may result in what we call a segmentation fault which means that there had been a write access error as the content is in read-only memory. It will inevitably lead to the crashing of the program. This type of string is called a "string literal ".
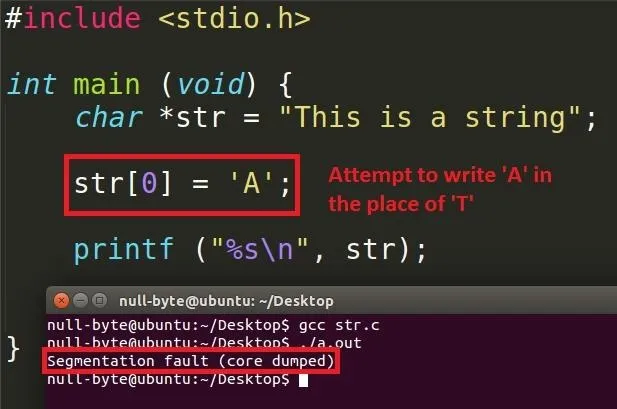
You'll find that it's possible to reference an element with the character pointer variable just like an array.
The String Library
The string.h header file contains prototypes (will explain this on a tutorial on functions) of many string manipulation functions such as string comparisons, copying strings and concatenating strings. If you're interested, head to Google, search it up and see what you can find.
Conclusion
Have a look over arrays and strings again, get familiar with how they work, especially the material I covered on memory in the last tutorial. When we go over buffer overflows, we will be analyzing the contents in memory and seeing exactly what is happening under the hood.
dtm.
Comments
Be the first, drop a comment!