NOTICE: Ciuffy will be answering questions related to my articles on my behalf as I am very busy. Hope You Have Fun !!!
Hello Guys,
Welcome to my first tutorial and in this tutorial we are basically going to create a port scanner in python ( I guess without external libraries ).
Before we starting build the project, I would first like to thank the null-byte community for been such a great help in my life: The fascinating website, Loving and always willing to help/learn members, selfless admin. You guys are awesome.
Aside my gratitudes, I would like to brief a little bit on networking.
INTRODUCTION TO THE WORLD OF NETWORKING
I am only going to treat what is needed to build this project.
From our friends at: WikiPedia
A port is a place where information goes into and out of a computer.. ( Read More )
A port scanner is a software application designed to probe a server or host for open ports. This is often used by administrators to verify security policies of their networks and by attackers to identify running services on a host with the view to compromise it.
A port scan or portscan can be defined as a process that sends client requests to a range of server port addresses on a host, with the goal of finding an active port. While not a nefarious process in and of itself, it is one used by hackers to probe target machine services with the aim of exploiting a known vulnerability of that service. However the majority of uses of a port scan are not attacks and are simple probes to determine services available on a remote machine.
To portsweep is to scan multiple hosts for a specific listening port. The latter is typically used to search for a specific service, ( For example, an SQL-based computer worm may portsweep looking for hosts listening on TCP port 1433 eg. SQL Slammer ).
WHAT WE MOSTLY USE TODAY ( TCP/IP )
The design and operation of the Internet is based on the Internet Protocol Suite, commonly also called TCP/IP. In this system, hosts and host services are referenced using two components: an address and a port number. There are 65536 distinct and usable port numbers. Most services use a limited range of numbers.
Some port scanners scan only the most common port numbers, or ports most commonly associated with vulnerable services, on a given host.
TYPES OF SCANNING PROTOCOLS
Lets quickly brush through some types of network scanning protocols.
TCP Scanning
The simplest port scanners use the operating system's network functions and are generally the next option to go to when SYN is not a feasible option ( described next ). Nmap calls this mode connect scan, named after the Unix connect() system call. If a port is open, the operating system completes the TCP three-way handshake, and the port scanner immediately closes the connection to avoid performing a Denial-of-service attack. Otherwise an error code is returned. This scan mode has the advantage that the user does not require special privileges. However, using the OS network functions prevents low-level control, so this scan type is less common. This method is "noisy", particularly if it is a "portsweep": the services can log the sender IP address and Intrusion detection systems can raise an alarm.
SYN Scanning
SYN scan is another form of TCP scanning. Rather than use the operating system's network functions, the port scanner generates raw IP packets itself, and monitors for responses. This scan type is also known as "half-open scanning", because it never actually opens a full TCP connection. The port scanner generates a SYN packet. If the target port is open, it will respond with a SYN-ACK packet. The scanner host responds with an RST packet, closing the connection before the handshake is completed. If the port is closed but unfiltered, the target will instantly respond with a RST packet.
The use of raw networking has several advantages, giving the scanner full control of the packets sent and the timeout for responses, and allowing detailed reporting of the responses. There is debate over which scan is less intrusive on the target host. SYN scan has the advantage that the individual services never actually receive a connection. However, the RST during the handshake can cause problems for some network stacks, in particular simple devices like printers. There are no conclusive arguments either way.
UDP Scanning
UDP scanning is also possible, although there are technical challenges. UDP is a connectionless protocol so there is no equivalent to a TCP SYN packet. However, if a UDP packet is sent to a port that is not open, the system will respond with an ICMP port unreachable message. Most UDP port scanners use this scanning method, and use the absence of a response to infer that a port is open. However, if a port is blocked by a firewall, this method will falsely report that the port is open. If the port unreachable message is blocked, all ports will appear open. This method is also affected by ICMP rate limiting.
An alternative approach is to send application-specific UDP packets, hoping to generate an application layer response. For example, sending a DNS query to port 53 will result in a response, if a DNS server is present. This method is much more reliable at identifying open ports. However, it is limited to scanning ports for which an application specific probe packet is available. Some tools (e.g., nmap) generally have probes for less than 20 UDP services, while some commercial tools (e.g., nessus) have as many as 70. In some cases, a service may be listening on the port, but configured not to respond to the particular probe packet.
ACK Scanning
ACK scanning is one of the more unusual scan types, as it does not exactly determine whether the port is open or closed, but whether the port is filtered or unfiltered. This is especially good when attempting to probe for the existence of a firewall and its rulesets. Simple packet filtering will allow established connections (packets with the ACK bit set), whereas a more sophisticated stateful firewall might not.
Window Scanning
Rarely used because of its outdated nature, window scanning is fairly untrustworthy in determining whether a port is opened or closed. It generates the same packet as an ACK scan, but checks whether the window field of the packet has been modified. When the packet reaches its destination, a design flaw attempts to create a window size for the packet if the port is open, flagging the window field of the packet with 1's before it returns to the sender. Using this scanning technique with systems that no longer support this implementation returns 0's for the window field, labeling open ports as closed.
FIN Scanning
Since SYN scans are not surreptitious enough, firewalls are, in general, scanning for and blocking packets in the form of SYN packets.3 FIN packets can bypass firewalls without modification. Closed ports reply to a FIN packet with the appropriate RST packet, whereas open ports ignore the packet on hand. This is typical behavior due to the nature of TCP, and is in some ways an inescapable downfall.
Other Scan Types
Some more unusual scan types exist. These have various limitations and are not widely used. Nmap supports most of these.
X-mas and Null Scan - Similar to FIN scanning, but:
X-mas sends packets with FIN, URG and PUSH flags turned on like a Christmas tree.
Null sends a packet with no TCP flags set.
Protocol Scan - Determines what IP level protocols (TCP, UDP, GRE, etc.) are enabled.
Proxy Scan - A proxy ( SOCKS or HTTP ) is used to perform the scan. The target will see the proxy's IP address as the source. This can also be done using some FTP servers.
Idle Scan - Another method of scanning without revealing one's IP address, taking advantage of the predictable IP ID flaw.
Cat Scan - Checks ports for erroneous packets.
ICMP Scan - determines if a host responds to ICMP requests, such as echo (ping), netmask, etc.
What we basically need to know is the TCP/IP Protocol.
SCREENSHOT OF FINAL WORK
SCRIPT
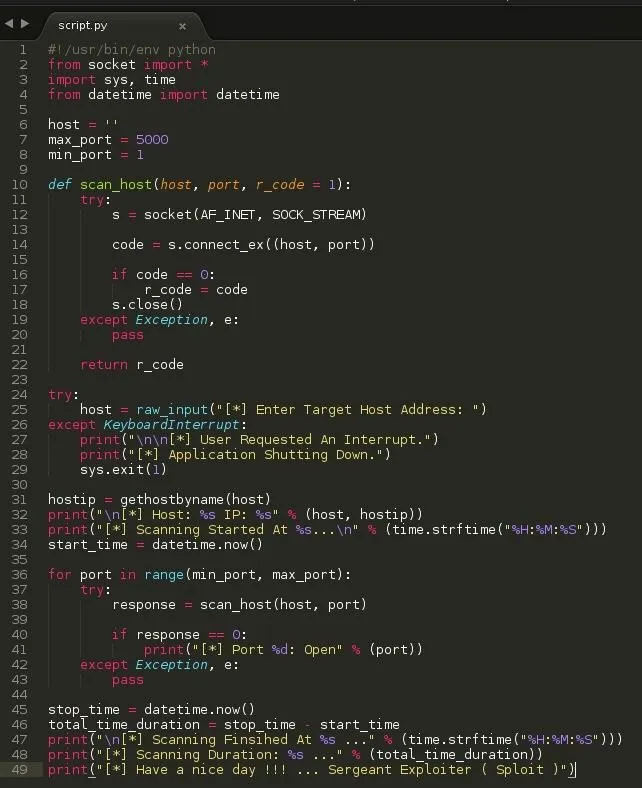
BUILDING THE PORT SCANNER
Our port scanner is going to be a simple one, Less than 50 lines and the codes used are basic.
Let's begin.
SECTION 1: IMPORTING MODULES
Socket - Important ( 10 / 10 )
Datetime - Optional ( 1 / 10 )
Sys - Maybe Important ( 5 / 10 )
Time - Optional ( 1 / 10 )
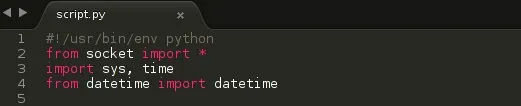
from socket import * - From the socket module, import everything
import sys, time - Import the sys module, Import the time module ( We can import as many modules as we want so far as we put a comma in between them )
from datetime import datetime - From the datetime module, import function datetime and leave the rest
SECTION 2: DECLARING PROGRAM SETTINGS
Basically, the settings we need are the host address , start and stop port ( Can be embedded in the script, But we wanna give others the chance to change it with ease should our script get out. )

Our script has been limited to port 5000 for quick demonstration.
SECTION 3: INITIATING PROGRAM
Lets ask the user for the target host address, This could be a url address of the host or the direct numberic ip address.
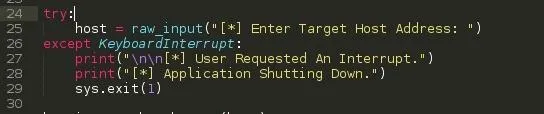
Except KeyboardInterrupt
This handles keyboard interrupts ( Ctrl + C ) should the user want to close the script for whatever purposes. This prevents python from stopping our program execution and spilling its Keyboard Interrupt exception code to the screen. ( Kind-off seems unprofessional ). Like this ...

Let's see what our program does when a user hits the interrupt command.
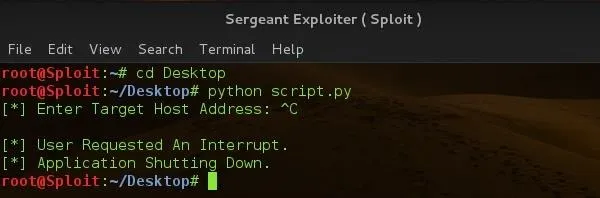
Nicely done !!!
SECTION 4: GETHOSTBYNAME

This function simply returns the ip numberic values of a host address or url.
When we call this function, we add our host address or url in open brackets and maybe associate it with a print like the screenshot which should echo the ip address of the host url or hostname.
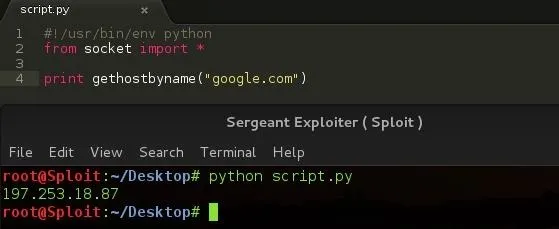
It prints out the ip address of Google.
SECTION 5: DATETIME
This function simply returns the current time value of the OS.
SECTION 6: SCANNING
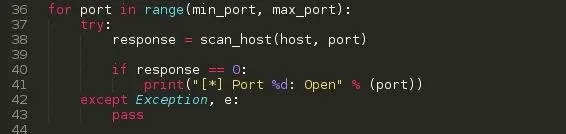
min_port - 1
max_port - 5000
range - It generates a list of numbers, which is generally used to iterate over with for loops. It takes two args, min number and max number. ( Read more on Range()
for port in range(min_port, max_port): For each number alias port in range, do something.
Now the try and except statment ensures the program execution is not stopped by an error ( except statement catches the error ).
In the try statement, response is calling a function scan_host and this function is taking two arguements: host and port ( We will explain the function in the next section). Response is a variable that holds the returned value from the scan_host function.
If the received value from scan_host is 0, print out Port <port number>: Open ....
When an exception or error occurs, pass simply allows the script to continue its execution.
SECTION 7: SCAN_HOST FUNCTION
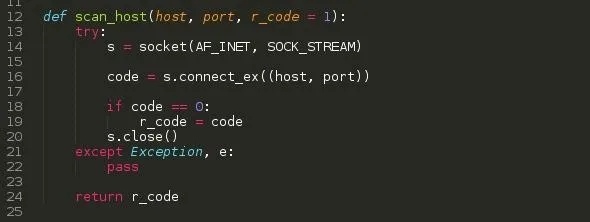
This basically utilizes the OS socket function to connect to the intended target. It accepts two arguements ( Actually 3 but r_code is optional and has been set to 1 ).
s - Initiates the sockets
code - Executes the connect function and captures the connection result ( Whether successful, failed )
if the code value is 0 which is what most linux programs use when there is successful execution, r_code is set to code which is 0.
The socket is then closed, Should an error occur: The except function is executed and pass allows the program to continue execution. ( Remember: r_code will still be set to 1, when the response variable receives it: It won't do anything since it has no command for 1 )
return - Simply returns r_code to its caller
NOTE: For the script to print out an open port, when r_code is set to 0 and the response variable receives it then we can have an open port printed out.
SECTION 8: CONCLUSION
I've successfully explained the needed part of the script and now it's time for testing ...
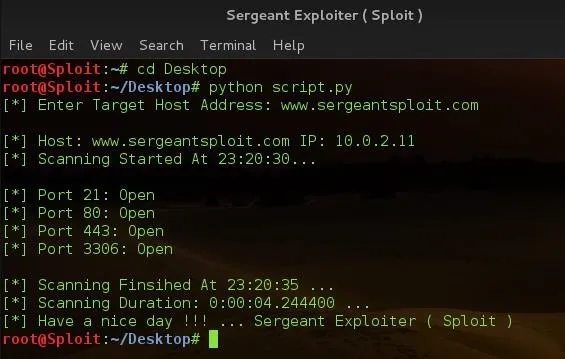
Let's test nmap to see it's result
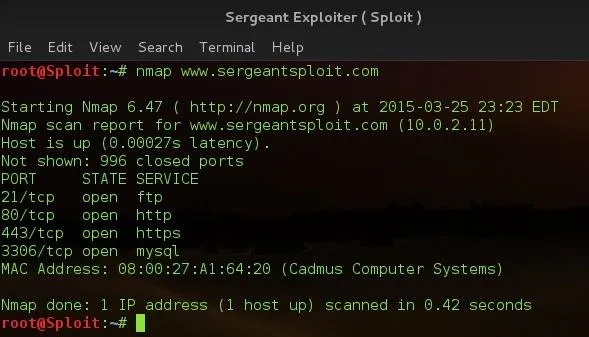
i think we created our own nmap ( Don't let this get into your head, Nmap is way advanced than our script ).
NOTE TO ALL
www.sergeantsploit.com is not a domain, I modified my /etc/hosts file to demonstrate the gethostbyname function. As you can see, its ip is 10.0.2.11 which is part of my internal NAT Network Adresses. Please don't go scanning ghost domains. :)
IN A NUT SHELL
Hope we had fun and notify me of any misinformation, typing errors or anything that needs correction. Have a nice day !!!!
# Sergeant
Comments
Be the first, drop a comment!