Welcome back! In the last training session we covered how to write and execute scripts. But today we'll be getting back to the code!
Today we'll be covering something that is extremely important for building real-life applicable scripts, file input and output. When I say "...file input and output.", I mean reading from and writing to files. This is an extreme step for making our scripts applicable.
We'll start by covering our two main file I/O modes, then we'll cover manually opening/closing files, and finally we'll cover automating the open/closing process. So, let's get started!
The Two Main Modes
There are many modes that we can use when performing file I/O. However, there are two modes that stand to be used more often than any others. These file modes are the basic read ('r') and write ('w').
The 'read' mode is represented by the single character string 'r'. Whereas the 'write' mode is specified by the equally lengthy string 'w'. These modes let us read and write to the file(s) we choose.
Note: The standard write mode will re-write everything in the file, we'll cover how to avoid this in a later, more advanced article.
Now that we know our two main modes and what they do, let's move on to actually reading and writing!
Manual File I/O
When we're performing I/O on files, we choose to open and close them manually. This method of opening/closing is not recommened, as failure to properly close a file can lead to some serious errors. While it is not recommended, it's a good introduction to the syntax for file I/O. So let's get started!
We'll start by making a file and putting some text in it. We'll be making a file named "file" and we'll simply be putting the words "Hello, Null Byte!" inside. Let's do that now:
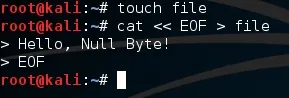
Now that we have our file, let's fire up our interpreter and do some I/O!
We'll be reading the file first, then we'll re-write it. To start, we need to actually open the file. This creates an object, so we'll need to store it in a variable. We'll be naming our file variable 'file' for simplicity's sake.
When we open a file, we use the open() function along with giving the path of the file as a string and the mode we'd like to use as arguments. Let's see an example of this syntax and open up our file for reading:

We can see here that we've successfullly opened our file in read-only mode. Let's go ahead and read the contents by printing the results of using the .read() method on our file object:
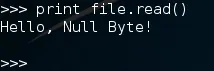
We can see here that it has printed the files content along with a newline (\n). Now that we've completed our reading, we need to close the file. This is extremely important, if we don't close the file it will cause errors and could possibly corrupt the file. We can close files using the .close() method on our file object. Let's go ahead and close our file now:

There, we successfully opened, read, and closed our file. Now let's speed through the process again, but this time we'll be writing to the file.
Note: When using write mode, you write to the file using the .write() method. Also, you must manually enter newlines into the file.
Let's see what it would look like if we manually wrote 'Hello, world!' into our file:

We can see here that we opened our file into write ('w') mode, wrote the string "Hello, world!" to it (manually adding a newline), and we closed the file. Let's read our file from the terminal to see if our changes we're successful:
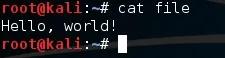
Alright, now that we know how to manually open and close files, let's try and automate this.
Automated File I/O
When I say automated, I mean that we don't have to manually open and close our files. We can use with and as to open and close our files for us, this way we don't have to risk file corruption.
First, we must enter with followed by our standard open() function in whatever mode we want (we'll be using read mode), then we enter as, and finally we enter the alias of our file. The alias would serve as the temporary variable name for our file. We end this with a colon and move on!
Let's see with and as in action:

Now you may notice that the prompt has changed. When we use with and as, we must place all of our I/O in the body of our with/as statement. Let's go ahead and read our file, then we'll execute this block of code by hitting enter twice:
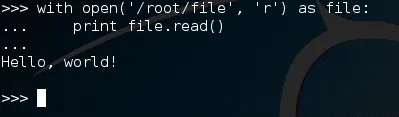
Here we can see that we we're able to print the content of our file without having to open and close our file manually! This can be very useful by removing the need to open and close files manually.
Let's go ahead and use this new automatic method to write to our file. We'll make this quick because we've already covered all of this:

Now let's read our file and see if it was written successfully:
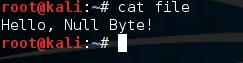
There we go! Let's wrap this up shall we?
Wrapping It Up
We learned a lot of very practical stuff here today, so I hope you all understood. Today we learned how to perform basic file input and output. We'll move on to the advanced file I/O topics in due time.
If you have any questions or concerns, leave them below and I'm sure they'll be addressed!
Thank you for reading!
-Defalt
Comments
Be the first, drop a comment!