Welcome back everyone! It's been quite a while hasn't it? Today we're going to kick off the second part of the Python training series by introducing modules. We'll start off by explaining what a module is and we'll give an example. So, let's get started!
What Are Modules?
Modules are very important for making specialized code. A module is a set of functions and/or classes that have been pre-built to perform certain tasks.
You might be asking, "Why keep everything separate?". Think about it this way. If you've ever been camping (No I'm not talking about CoD) you'll know that in order to properly set things up, you need to bring the right equipment.
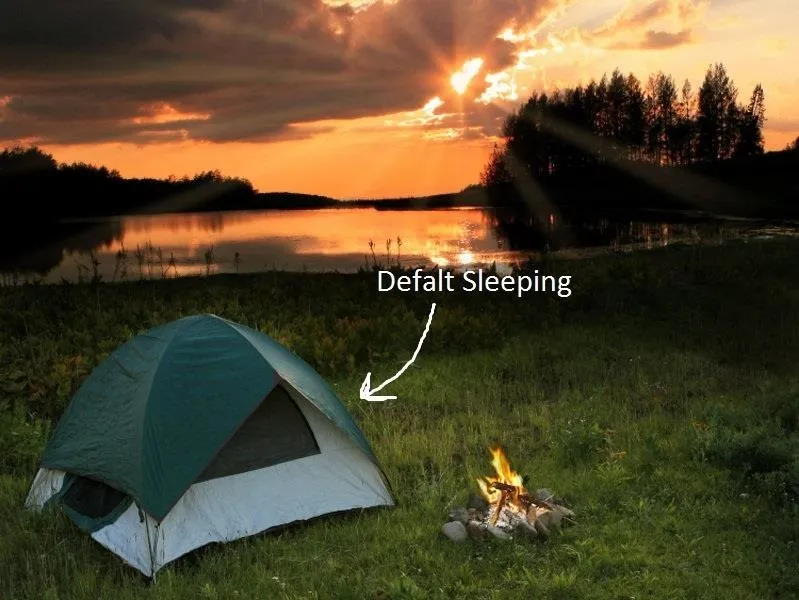
Now imagine if you were camping in a specific environment, like Alaska. You'd need special equipment to camp there. Imagine if you had to bring every single piece of equipment for every single environment with you every time you camped. It would be a huge pain! The same goes for Python, why keep all the functions and classes in one place? It'll do nothing but slow the interpreter down. If we keep all the code separated into groups, it'll be easier and faster to just pick out what we need and run with it!
Now that we have an idea of what modules are, we can demonstrate how to use them. For this example, I've made a module named trainingExample (available here). Let's get to it!
Import the Entire Module
When we want to use a certain module, we need to import that module. When we import something, we're bringing it into our space so that we can use it's functions and such. We can import entire modules, or we could import specific functions out of them. We'll be performing both.
We import modules using the import keyword (shocking, right?). We follow this keyword with the name of the module we want to import. Let's import our entire module now:

There we go. Now that we have our module imported, let's call the hello() function out of it. If we import entire modules, we need to designate the module name before calling the function so the interpreter knows where to look for that function. Let's go ahead and call our hello() function out of our module:
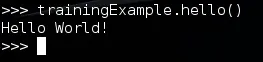
Alright, we were able to import the entire module and call out our function specifically! Now that we've done it this way, let's try to import the hello() module only, without the other functions.
Specify the Functions and Classes
An alternative to importing entire modules, we can explicitly state which functions and classes we'd like to have. This will bring them into our space just as if we created them.
When we want to import specific things, we use the keyword from. We tell the interpreter "From this module, I want to import this function". We start with the keyword from, followed by the name of the module to import from. Then we list the functions/classes we'd like to import. If you wish to import more than one object, you must separate them by commas.
Now that we've covered how to import specific objects, let's explicitly import our hello() function:

There we go. Now that we've imported the only the hello() function, we can call it without specifying the module. Let's go ahead and call our function:
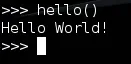
Alright! That finishes method number two, now let's wrap things up shall we?
Wrapping It Up
We covered some very important topics here. In order to use modules properly, we need to know how they work and what exactly they are. Today we covered the basics of importation and the keywords associated with it. Next time, we'll get into one of the popular standard modules.
Thank you for reading!
-Defalt
Comments
Be the first, drop a comment!