In the last iteration of how to train your python, we covered basic string manipulation and how we can use it to better evaluate user input. So, today we'll be covering how to take user input.
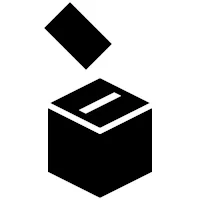
User input is very important to scripting. How can we do what the user says if we can't tell what the user wants? There are multiple ways to take input, we can give the user a prompt and take input from them directly, or we could use flags/switches, and take their input before the script is even executed. We'll be taking user input directly today, but don't worry! We'll cover flags/switches later! So, let's get started!
Python's Input Function
Python provides us with nearly everything we'll need in order to build the best scripts we can, so naturally it provides us with a way to take user input.
We will be using the built-in function of "raw_input()" to take the user's input. It used to be that you had to use the "input()" function and then manually evaluate it, but raw_input() does everything for us!
Taking the Input
To start, we'll just be using the function without anything extra, just to get a feel for it. So, let's enter "raw_input" into our python interpreter...
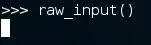
Now when we entered it, we were prompted with a blank line, this is the default prompt for this function. So, let's give it some input and see what happens...
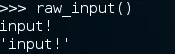
Now we've entered "input!" as our input, and it simply returned what we entered. Now you may be wondering if there is a way to give a prompt for the user, instead of just a blank line, well the answer is a resounding yes.
Giving the User a Prompt
When we take input, we need to ask the user something, instead of just providing them with a blank line. This is what the argument for the raw_input() function is, if we give it a string as an argument, it will display that string as a prompt. Let's see this is action...

Now we gave the function the string of "Give me your input!" and instead of displaying a blank line, it prompted the user with the string we gave it. We can use this to ask the user questions, so they know what they're supposed to be entering.
Returning the Result
In the first section of this article, we saw that the raw_input() function returned whatever string we entered. We can use this to set whatever the user enters as the value of a variable. To do this, we simply assign the raw_input() function to a variable, like so...
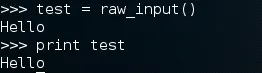
Here, we set the test variable equal to the raw_input() function with no arguments, then we entered "Hello" as our input, and printed the new value of test, which is now equal to what we've just entered.
Putting It All Together
Now that we've covered the individual aspects of taking input, let's put them all together and demonstrate what we've covered. We'll ask the user for input, with a prompt, and assign the result to a variable...

As we can see, I prompted myself for my name and where I'm from. I answered with "Defalt" and "Null-Byte". Now that we have the answers we wanted, let's relay them back to the user with a print statement...

Now we've fashioned a print statement using the what the user gave us as input. In a real scripting scenario, we could do things like take an IP address for a target, or have the user make a critical decision as to the function of the script.
There are many things to do with input, but now we know how to take it! This is an import first step in being interactive with the user. We'll cover flags/switches as input later in the series, but regular input should suffice for now.
The Exercise
Take your own input! Don't be afraid to experiment and make sure you leave your resulting code in the comments section! I might even copy-paste them and answer them! Remember to utilize what we covered in the last article while taking your input!
Feedback!
I'm really happy that we're finally back on schedule for the release times of these articles, I was a bit strapped for time recently but I'll try harder to clear some space in the future! I'll see you for your python's next training session!
Thank you for reading!
-Defalt
Comments
Be the first, drop a comment!