In the last iteration of how to train your python, we covered the concept of control flow and covered the booleans associated with it. Today, we'll be putting those to use as we discuss how to use if, else, and how to make conditional statements. So, Let's get started!
Conditional Statements
We'll be covering conditional statements first because we'll be using them with if and else. A conditional statement is simply a statement that returns True or False, all of the booleans we practiced in the last article were conditional statements. I strongly suggest that if you haven't read the last article that you do so before we continue, as it will be confusing without the information covered there. But, I'll show some example conditional statements so we can have a general feel for them...
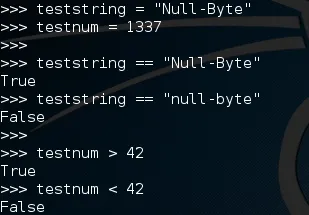
These are just some simple conditional statements to be used as examples, we should have a good grasp of conditional statements by carrying over what we learned in the last article. So now that we've covered conditional statements, let's get to if and else!
If and Else
Now when we want to implement some control flow, we use if and else. These let us test for certain conditions and take whatever actions we see fit based on the result. Here, we'll be taking input, and giving feedback based on the what the input was. We'll start by taking the input...

Here, we've simply asked the user where the best place to learn how to hack is. The answer (obviously) is Null-Byte. Now that we've taken our input, let's build our if statement and give the user a response...

The syntax for if statements is as follows. The word if, followed by our conditional statement, and then a colon. Once we've typed this line we press enter/return and then we can enter the actions to take if our condition statement equates to true. Be careful! The resulting actions to take must be one tab in from the if statement (This tells python that these are the actions to take). Once we've made our if statement and result, we make our else. If the input the user gave us isn't what we tested for, nothing happens. Else is a catch-all if something didn't meet our requirements. Now that we've built our if and else, let's see the output...

Alright, our if statement worked! But now let's test if the else works, let's re-assign the input value with more input and change what we give it...

Now, using the same if and else, let's see what the result would be...

There we go! Our if and else works like a charm! If the user inputs Null-Byte, it responds differently than anything else.
This is great, but what if we want to test for another condition if it doesn't meet the initial if criteria? We can use elif. Elif is simply short for else-if and this let's us test for another condition after we've used our initial if statement. We'll start by changing the input variable and giving it different input again...

Ok, so now that we've entered "incybernews" as our input, let's change the if and else block to test for another condition...

The if and else block is the same except for the addition of our new elif statement in the middle. Now that we've re-built our if and else block to accommodate the elif statement, let's run it and see what the output is...

There it is! Our elif statement caught that we entered incybernews after it passed through our initial if statement!
A Side Note
The reason behind using elif instead of more if's is because you can only have one if and one else, but you can have as many elif's as you need. We'll use this if we have to test for many different conditions at once.
The Exercise
Make your own if/elif/else blocks! Try and incorporate what we've covered up till now in this series in your code! Remember to leave your resulting code in the comments below!
Feedback!
Well, there we have it. If, elif, and else. These are some of things we'll use the most frequently throughout our scripting careers. Make sure to come back and join us for the next iteration of how to train your python!
Thanks for reading!
-Defalt
Comments
Be the first, drop a comment!