In the last iteration of how to train your python, we covered if/else and conditional statements. We're going to diverge a little today and cover lists. Lists are simply that, lists. It is a set of values located under one variable. Now that we know the definition of a list, let's learn about them!
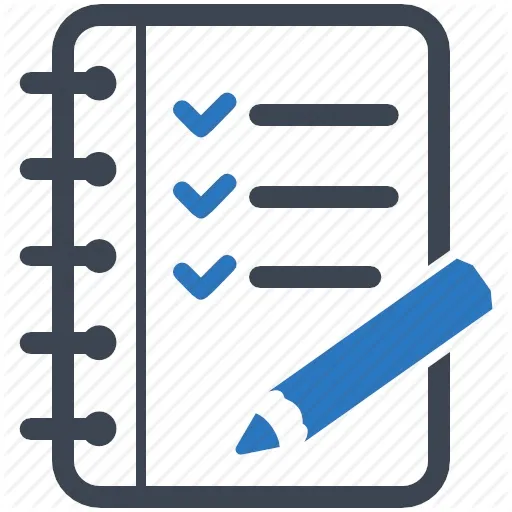
List Basics
Usually when we assign a value to a variable, we can only assign one per variable. A list let's us assign multiple values. We create a list by placing our set of values inside of brackets ([]) after we name our variable. When we put our values inside the brackets, they have to be separated by commas. Let's use this new information and create our first list!

We've set our variable name to "test" and have placed three values inside of our brackets, "Null", a single hyphen, and "Byte". Now that we know what lists are and how to make them, let's move on to indexing.
Indexing
You may be asking, "if there are multiple values, then how do I decide which one to use?". Well this is where we introduce indexing. Indexing is simply how to identify which element from our list we'd like to use. The way to call that element out of the list is by entering the list name followed by the element's index number in brackets.
There is a slight catch when it comes to indexing. We have to start counting at 0. I know it sounds weird, but eventually it'll become second nature. Remember when we made our test list? We placed three values inside of it, "Null", "-", and "Byte". That means that if we wanted to call "Null" out of the list, we'd have to enter test[0] as "Null" is the first element in the list. Let's call our elements out of the list one at a time using their index numbers...
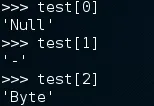
Here we see a good example of calling elements. By entering the name of the variable, in this case "test", followed by the index number of the desired element in brackets. We can use these for a multitude of things, but there are so many that we'll have to cover it later. Let's combine our calls into a print statement and combine them into the word they form...

Alright. Now that we've covered indexes and how to retrieve elements from a list, let's cover some basic methods.
Basic List Methods
If you remember from the string manipulation article, we covered methods. These are simply things we can use to do what we want. If you haven't read the strings article, I highly suggest it so that you can understand the concept of methods.
There are plenty of list methods, but today we'll be covering the three I use most often. These are...
- .append()
- .insert()
- .remove()
We'll start with .append(), and work our way down the list.
So, what .append() does is it places whatever we give it as an argument into the last place on the list. Let's reset our "test" list to be blank, we can do this by setting it equal to empty brackets, like so...

Now that our test list is empty, we can demonstrate .append() to it's full potential. When we try to use list methods, we simply enter the list name, followed by the method we want to use, along with the arguments inside of the method's parentheses. We'll be appending the integer 1 to our list...
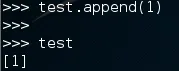
As we can see above, we used the .append() method with the argument of 1 on our test list. Then after we viewed the contents of test again, we can see that 1 has now been appended. But to see how append really works we'll have to put another value in and see where is it placed...

Now that we've entered our second append, we should be able to see the integer 2 in our list. Can you guess where it will be? Let's find out!

We can see above that when we call to see our list's contents again, the integer 2 has been placed after the 1. This is what differentiates append from the next method, insert.
When we used append, it placed our argument value at the end of the list. But if we want to decide where we place something, we use .insert()
The .insert() method takes two arguments. The first being where you would like to place the new element, and the second being the element you'd like to add. Let's add the third number to our list using the .insert() method...
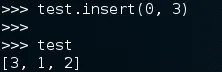
In the above example we added the integer 3 to the 0th index. This placed it before the number 1. This is the advantage of .insert() over .append(). With insert, you can decide where the element goes. Oops! It seems we've placed our numbers out of order, but don't worry! We can use the .remove() method to fix that right up!
The .remove() method, as you may have guessed, removes elements from a list. To use this method, we enter the name of our list, followed by our method and the element we'd like to remove as the argument. Let's use the .remove() method and get rid of that pesky 3...
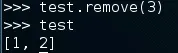
We called our method on test and asked it to remove the element 3. Then we called to see the contents of test again, and as we can see, the number 3 is gone! That concludes our basic list methods, so let's get on to the exercise!
The Exercise
Make and call elements from you own list! When/if you do these exercises, remember to incorporate what we've already covered into your code, that way you don't get rusty! Also, as always, don't be afraid to experiment!
Feedback!
Well, sorry for the dry spell, I've been working on a much bigger project. No spoilers, but I'm very excited about it, and I hope you will be too! The project will be announced as soon as we have a working prototype. As always, I'll see you for your python's next training session!
Thank you for reading!
-Defalt
Comments
Be the first, drop a comment!