NOTICE: Ciuffy will be answering questions related to my articles on my behalf as I am very busy. Hope You Have Fun !!!
Hello Guys,
Welcome to my tutorial and in this tutorial, We are basically going to
create a python script that serves as a proxy server ( Without External Libraries ).
I made this script when I had some knowledge of python sockets and also thought it was a cool thing to do. I want to share it with the community.
Before I begin, I would first like to thank the members who gave me positive feedbacks on my How To Make A Python Scanner Article, I am very grateful for your appreciation.
Also, Lets quickly talk about Proxies before we proceed
any further to help others who might have heard or read the name
somewhere but don't have a slightest idea of what it means.
INTRODUCTION INTO THE WORLD OF PROXIES
From WikiPedia - Proxy Server.
In computer networks, A proxy server is a server ( A computer system or
an application ) that acts as an intermediary for requests from clients
seeking resources from other servers. A client connects to the proxy
server, requesting some service, such as a file, connection, web page,
or other resource available from a different server and the proxy server
evaluates the request as a way to simplify and control its complexity.
Proxies were invented to add structure and encapsulation to distributed
systems. Today, most proxies are web proxies, facilitating access to
content on the World Wide Web and providing anonymity.
Lets see an illustration.
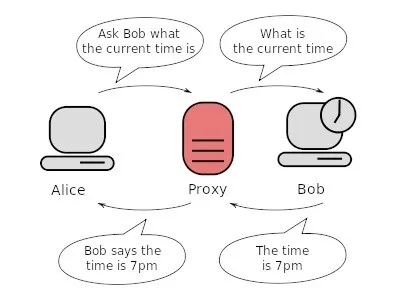
What Bob thinks is the server ( i.e the proxy ) asked for the current time, But what Bob didn't know was, Alice asked for the current time but through the proxy server. The proxy server returns the current time to Alice. So we can basically say, Server Bob has been tricked. The proxy server acts as a man in the middle serving two people without revealing their identities to each other, Each person sees only the proxy but not the other end.
While a proxy might seem straightforward to the typical end user, there are many variations of the simple proxy website. While you might want only you chat with Facebook friends while at work and need to hop online anonymously through a proxy, what's happening behind the scenes can be quite complex. While many proxies look similar on the user's end, they can be very different to the developers and programmers.
Note: There Are Many And Countless Ways To Detect Proxy Clients
There are many types of proxies of which I won't be listing. Here are the most basic and commonly used ones.
TYPES OF PROXIES
From ProxySites and WikiPedia:
PHP Proxy – A PHP proxy uses a script written in PHP to take webpages from your regular server and then processes your search request to protect your main server. A PHP proxy can be a form of CGI proxy or simply a bit of code to attach to the URL as you type it in. PHP proxies can also be used by site and program developers to help cloak their original server to increase the effectiveness of web applications.
CGI Proxy – A CGI proxy allows you to search through the proxy site for the URL you'd like to reach. CGI proxies allow you to search anonymously by hiding your IP address behind the proxy site. Thanks to this simplicity and anonymousness, CGI proxies are the most common proxies for the typical internet user searching for a means to unblock a website or search anonymously.
HTTP Proxy – The HTTP proxy works with web browsers (HTTP clients) and servers that support HTTP. The HTTP proxy caches pages, or store them, for faster retrieval and are used occasionally to increase metadata services available on the website.
Gateway Proxy – A proxy that allows requests and information to travel through without modification is called a gateway, or gateway proxy. These can also be called tunneling proxies as they "tunnel" around blocks put into systems through filters. Tunneling proxies are used frequently in workplaces and educational offices where there are frequent blocks on material that employees or students wish to access.
Content Filter – The filter that might be blocking the sites you wish to access is likely a form of a proxy as well. These specialized proxies called content filters require all requests to pass through for review. The content filter proxy then blocks requests that match requests on a "black list" or based on certain security settings. Depending on the complexity of the content filter, network administrators can add to or remove items from the black list.
DNS Proxy - A DNS proxy server takes DNS queries from a ( Usually local ) networks and forwards them to an Internet Domain Name Server. It may also cache DNS records.
Anonymous HTTPS proxy - Users wanting to bypass web filtering, that want to prevent anyone from monitoring what they are doing, will typically search the internet for an open and anonymous HTTPS transparent proxy. They will then program their browser to proxy all requests through the web filter to this anonymous proxy. Those requests will be encrypted with https. The web filter cannot distinguish these transactions from, say, a legitimate access to a financial website. Thus, content filters are only effective against unsophisticated users.
Use of HTTPS proxies are detectable even without examining the encrypted data, based simply on firewall monitoring of addresses for frequency of use and bandwidth usage. If a massive amount of data is being directed through an address that is within an ISP address range such as Comcast, it is likely a home-operated proxy server. Either the single address or the entire ISP address range is then blocked at the firewall to prevent further connections.
Suffix proxy - A suffix proxy allows a user to access web content by appending the name of the proxy server to the URL of the requested content. Suffix proxy servers are easier to use than regular proxy servers but they do not offer high levels of anonymity and their primary use is for bypassing web filters. However, this is rarely used due to more advanced web filters.
TOR Proxy - Tor ( The Onion Router) is a system intended to enable online anonymity. Tor client software routes Internet traffic through a worldwide volunteer network of servers in order to conceal a user's location or usage from someone conducting network surveillance or traffic analysis. Using Tor makes it more difficult to trace Internet activity, including "visits to Web sites, online posts, instant messages and other communication forms", back to the user. It is intended to protect users' personal freedom, privacy, and ability to conduct confidential business by keeping their internet activities from being monitored.
"Onion routing" refers to the layered nature of the encryption service: The original data are encrypted and re-encrypted multiple times, then sent through successive Tor relays, each one of which decrypts a "layer" of encryption before passing the data on to the next relay and ultimately the destination. This reduces the possibility of the original data being unscrambled or understood in transit.
I2P anonymous proxy - The I2P anonymous network ( I2P ) is a proxy network aiming at online anonymity. It implements garlic routing, which is an enhancement of Tor's onion routing. I2P is fully distributed and works by encrypting all communications in various layers and relaying them through a network of routers run by volunteers in various locations. By keeping the source of the information hidden, I2P offers censorship resistance. The goals of I2P are to protect users' personal freedom, privacy, and ability to conduct confidential business.
Each user of I2P runs an I2P router on their computer (node). The I2P router takes care of finding other peers and building anonymizing tunnels through them. I2P provides proxies for all protocols (HTTP, IRC, SOCKS, ...).
SOME USES OF PROXIES
We may all have our reasons for using proxies, But mostly, People use proxies for anonymous surfing, hacking D-Grade websites and playing other pranks on the web. Most common uses are as follows;
- Filtering of encrypted data
- Bypassing filters and censorship
- Logging and eavesdropping
- Improving performance
- Security
- Cross-domain resources
- Translation
- Anonymousity
Ok, I think that is enough for a brief introduction.
BUILDING OUR PROXY SCRIPT
The script is cross platform since the modules imported are cross-platform i.e available in both windows python and linux python.
SCREENSHOT 1: IMPORTING MODULES

import socket, sys - Import the socket module, Import the sys module. ( We can import as many modules as we want so far as we put a comma in between them )
from thread import * - From the thread module, Import everything ( functions )
SCREENSHOT 2: DECLARING PROGRAM SETTINGS
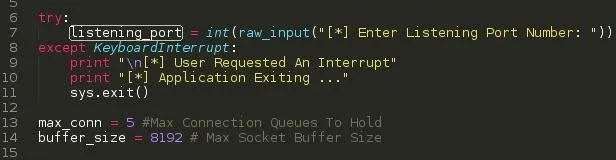
listening_port - We ask the user for a listening port using the raw_input function, After we assign the input to the listening_port variable.
max_conn - We limit the amount of connections to our proxy server.
buffer_size - Maximum socket buffer size ( Recommended: 4096 )
Except KeyboardInterrupt
This handles keyboard interrupts ( Ctrl + C ) should the user want to close the script for whatever purposes. This prevents python from stopping our program execution and spilling its Keyboard Interrupt exception code to the screen. ( Kind-off seems unprofessional ). Like this ..

Let's see what our program does when a user hits the interrupt command.
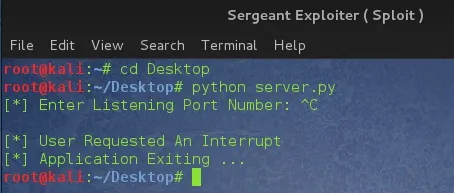
Nicely done !!!
SCREENSHOT 3: INITIATING THE PROGRAM
We are going to enclose all our codes in functions and later call them during our usage.
We are going to use three functions in the script.
Function 1: start() - Main Program
Function 2: conn_string() - Retrieve Connection Details
Function 3: proxy_server() - Make connection to end server
SCREENSHOT 4: Function: start()
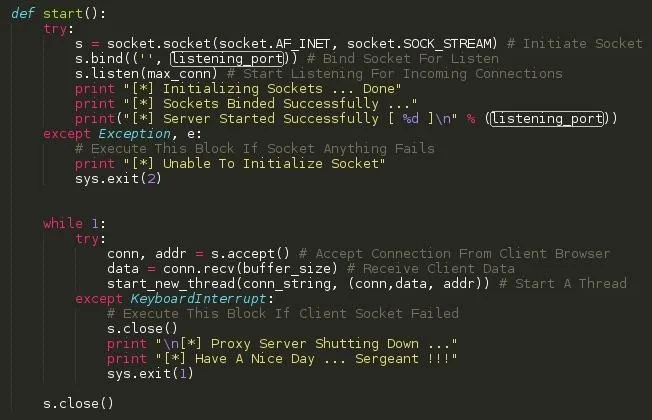
What this function does is to
1. Set up the socket
2. Bind the sockets
3. Start listening for incoming connections
When and error occurs, Print the custom message and exit the script
4. When a connection arrives from the client, accept it, receive the clients data ( Browser request ) and start a thread. The thread in-turns calls another function conn_string and pass three arguements:
- conn ( Connection Stream )
- data - clients data ( Browser request )
- address - ( Address from the connection )
When a user interrupts the program, the server connection stream closes, prints the custom message and exits the proxy script.
SCREENSHOT 5: Function: conn_string()
Arguements: conn, data, addr
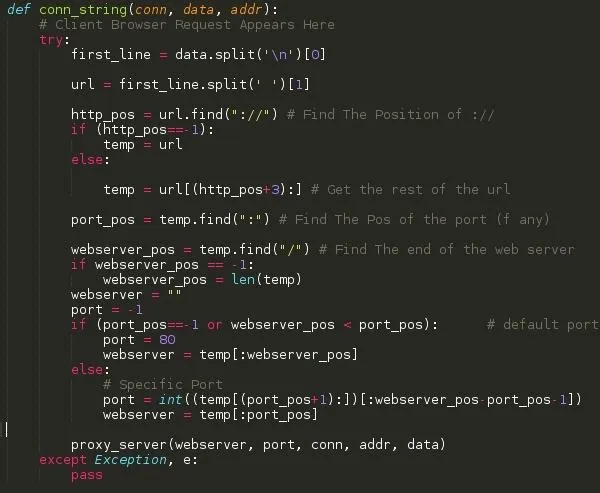
This is an example of a client browser request ( The connection is to Null Byte ).
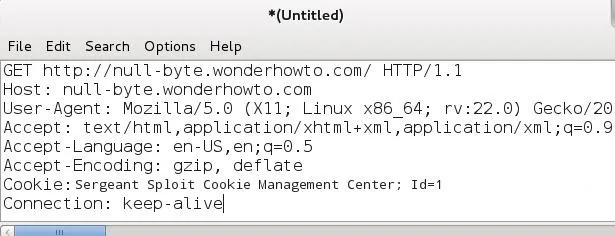
What this function conn_string basically does is to retrieve the host address, port (if specified by client) in the received client data, call another function proxy_server and pass five arguements to it.
webserver - host address
port - webserver port
conn - connection stream
addr - address from connection stream.
data - client browser data
SCREENSHOT 6: Function: proxy_server()
Arguements: webserver, port, conn, data, addr
NOTE: An error I made. The s.connect function in the fourth line in the image below should have the variable webserver instead of host. Failure to change will deny the user of relaying the information to the internet.
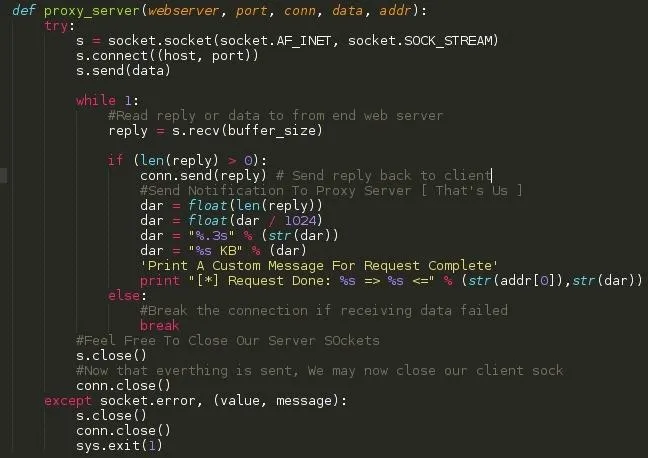
This function creates a new socket, connects to the webserver and sends the clients request, receives the reply, forwards it back to the client without modifying it, prints a custom success message and closes the streams.
SCREENSHOT 5: FINAL WORK
Let's make the magic work ... We simply type start() on a last line of the script.




What this does is to start the main program .... We now have a working python script .....
Not soooo fast ??? We haven't tested it. ( A connection to google )
To use the script, Simply set your browser proxy to 127.0.0.1 and desired port, Make sure your script is running on that port ( Mine is on port 8001 )
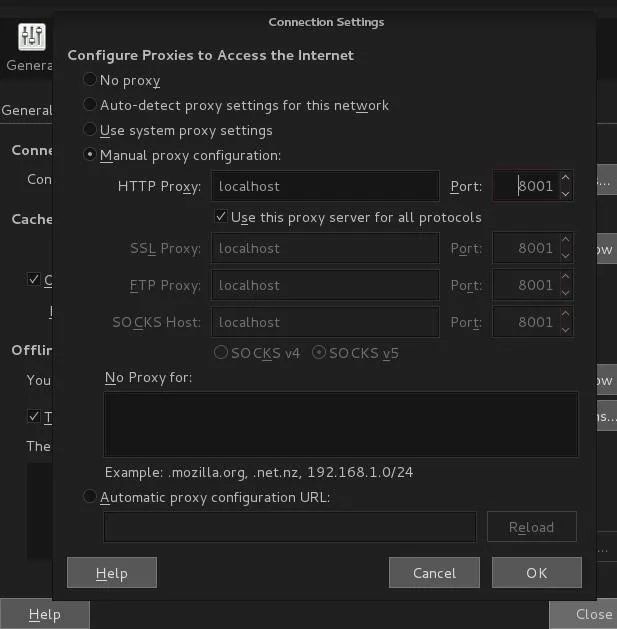
Test Run.
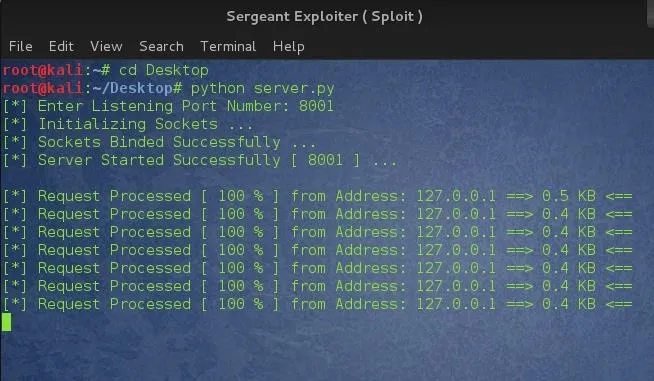
Ahh !! :) ......... Successfully working.
CONCLUSION
I hope we had fun and learnt something today. This script can be hosted online ( maybe on a vps ) to serve as a proxy and receive requests from clients. We can also make logs from the script right after sending the reply back to the client.
Notify me of any errors, misinformation or anything that needs correction. Hope to see you soon and Have a nice day !!!
# Sergeant
Comments
Be the first, drop a comment!