Welcome back! In the last iteration of how to train your python, we talked about functions, and we even made our own! We're going to move on to more types of data arrays (much like lists) in today's discussion.
We're going to be discussing tuples, which can be easily understood with a little thinking, then we'll be discussing dictionaries since we'll be on the topic of data arrays. If you haven't read the article about lists, I recommend you do so, otherwise tuples will be slightly confusing. So, let's get started with tuples!
What Is a Tuple?
A really simple way to think of this is that a tuple is just a list that can't be changed. If you read any documentation about tuples, it will say that they are 'immutable'. This means that they cannot be mutated or in other words, changed.
If you remember about lists, you'll know that lists are surrounded by brackets, tuples on the other hand are surrounded by parenthesis. We're going to be making a tuple and a list with the same values, and comparing them. Let's do that now...
Making Our Own Tuples
So, we'll make our tuple and list contain the same values, 'Null', and 'Byte'. Let's make our tuple...

Now let's make our list...

Now we can call these elements out the same, but we can't modify, or mutate, the tuple. Let's call the .append() method on our list and tuple to demonstrate this difference. First, we'll call it on the list, then we'll print the list...
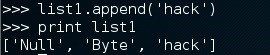
We can see here that we appended the work 'hack' to our list with no problem, now let's try and do the same with our tuple...

We can see here that we cannot modify our tuple. There isn't really much to do with tuples that can't be done with lists, but if you come across them in some code, you'll know what's going on. Now, let's move on to dictionaries!
What Is a Dictionary?
A dictionary is a set of keys and values. Think of it like a real life dictionary, there are words and definitions. The words serve as keys and they represent the definition.
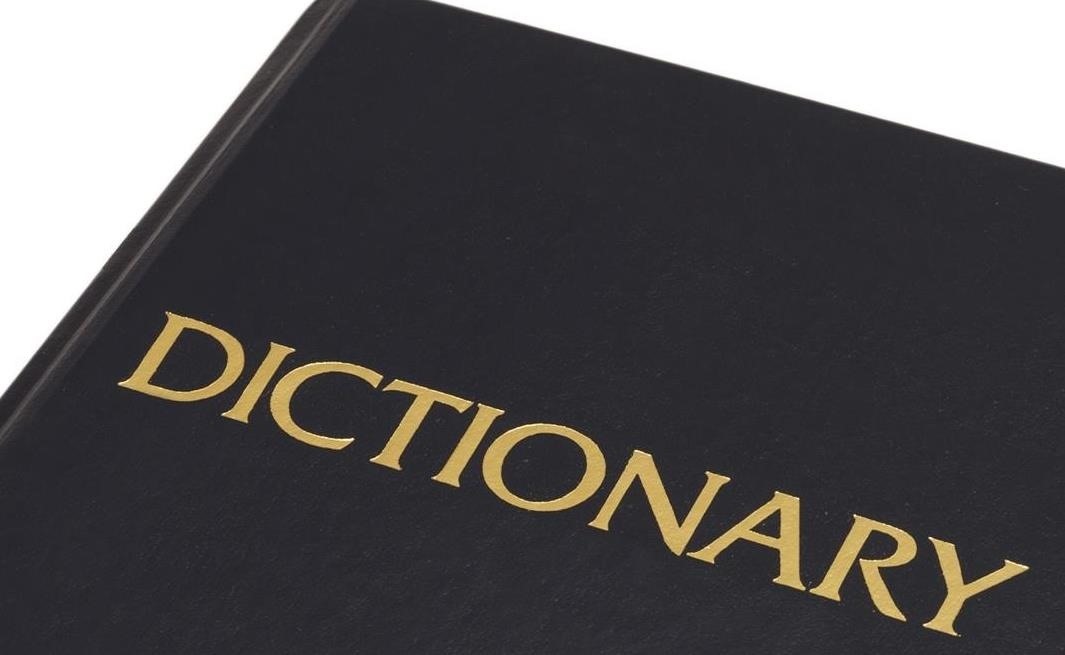
We can use dictionaries for a variety of things, such as storing user input in a very organized way, or extracting names and values out of a text file. Today, we'll just be building a simple dictionary and going over the three methods used to call out the keys and values.
Making Our Own Dictionaries
So, we'll be creating a dictionary with two keys, 'word1', and 'word2'. We'll give them the values of 'Null' and 'Byte'. Then we'll see the three different ways to present this information. So, let's start by making our dictionary...

The way we make dictionaries is we make our first curly bracket, then we put our first key. In this case, it's the string 'word1'. Now, when we want to designate the value for a key, we put the value we want after a colon. So, we've place 'Null' on the other side of the colon from 'word1'. Now that this key/value pair of the dictionary is complete, we put in a comma to separate it from the next pair, which is 'word2' and 'Byte'.
Now that we have our dictionary, let's use our three methods to see what's inside of it, our three methods are...
- .keys()
- .values()
- .items()
Let's use each of these and briefly discuss them. We'll start with .keys()...

We can see that .keys() shows us all of the keys in the dictionary, now let's move on to .values(), can you guess what it does?

As we can see, .values() shows us the values within the dictionary, who would've guessed? Now, let's move on to .items()...

Here we see that .items() shows us the keys and their values, not just one or the other. It's also worth noting that even though the dictionary itself was returned as a list, the pairs of items within the dictionary have been returned as tuples. Now, let's wrap this all up shall we?
Wrapping It Up
Today we covered tuples and dictionaries. There wasn't really much to say about tuples, but knowing what they are will help us to read other's code. Dictionaries however, can be very handy, and we'll definitely be covering them in more detail later!
Feedback!
Let me know what you think! Leave any questions you may have and I'm positive they'll be answered! I'm sorry about the dry spells, but this project has recently picked up momentum and is growing rather quickly! I think you'll all be really excited when it gets announced!
Thank you for reading!
-Defalt
Just updated your iPhone? You'll find new emoji, enhanced security, podcast transcripts, Apple Cash virtual numbers, and other useful features. There are even new additions hidden within Safari. Find out what's new and changed on your iPhone with the iOS 17.4 update.
4 Comments
I remember learning about tuples, and how confusing it was for me to tell the difference between tuples and regular lists. However, you made it easy to understand. Kudos for you!
(I'm very happy about this series)
nice job bro...thanks for the sharing +1
Hacked by Mr_Nakup3nda
So...Tuples are like permanent Lists that cannot be edited?
Yes.
Share Your Thoughts