Welcome back! In the last iteration of how to train your python, we covered loops, today we'll be covering something that I wish I had learned about much earlier in my scripting career, errors.
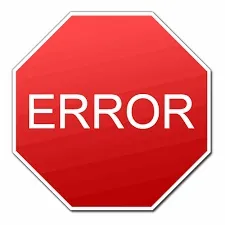
We all run into them, and they can be frustrating, but there is also a silver lining to them. Today, we'll be discussing why some errors are unavoidable, and what we can do when we run into them. Also, this article will be more of a concept and less of writing actual code, but the information is equally valuable. So, let's get started!
Errors Aren't Always Bad
A common misconception is that if an error occurs, you've done something wrong, this isn't always the case, sometimes errors can occur based on the user's input, or sometimes a process may fail, the point is that not all errors mean that something is broken, it just means that something needs to be accounted for. As a matter of fact, we can actually use errors to our advantage, but that is beyond the scope of this article. Now that we've conveyed the point that errors are eventually unavoidable, let's discuss how to diagnose an error.
Diagnosing an Error
When we run into an error, it will be rather obvious. I'm sure we've all seen those big chunks of text appear when when we mess something up. Errors will commonly look like this...

Now, since we have an error, we need to know what caused it. When we receive the error message, it will provide us with a line number, and the text that is on the offending line, a marker will also be placed at the point of failure. At the very bottom of the message, we see the type of error that occurred, in this case, it was a TypeError, since we tried to print a string and an integer together. There are many kinds of errors, and these errors are organized into a hierarchy. This hierarchy is outside the range of this article, so I'll just leave a link to it here.
Expecting Errors
In order to build the most solid script possible, we need to account for every possible error. It may seem hard to spot possible error points at first, but picking them out gets easier with practice. The main thing to remember in trying to find possible points of failure is that the user will cause more errors than anything. Invalid input is one the biggest causes of errors out there, right alongside faulty code itself.
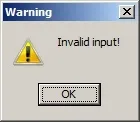
The point of this section is to remember to re-read your own code, very carefully. Once you've gotten good at spotting points of failure, you'll enter a new level of scripting skill. Now that we've learned to expect our errors, let's learn how to actually code for them...
Handling Errors
Alright, enough talking about concepts, on to the code! In order to handle errors, we use try and except. It's rather self explanatory when you think about it, try this, and give an exception to this error. Using try and except allows us to accommodate for errors that may occur.
In order demonstrate this, we'll be building a simple script to ask the user for a number, and repeat the number. But it will only repeat the number if the user enters an number, no words allowed. First, we'll build it without using try and except, then we'll incorporate them into our code and see the difference. So, let's start by building a version without try or except...
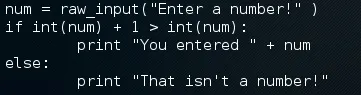
Alright, in the above screenshot we ask the user for a number, and then we attempt to test if it is a number by attempting to add one to it. If it works, we repeat the number to the user, if it doesn't, we tell the user that they did not give valid input. Let's see what happens when we input a number, then what happens when we input a string...
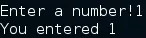

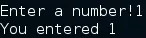

As we can see above, we get the proper output when we give a number, but when we give a string, it returns an error. This is because we can't make an integer out of a string, so let's rebuild our script, but this time we'll use try and except...
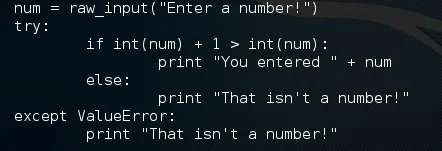
Now that we have our debugged code, let's run through it again and see if we can get it to return an error...
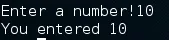

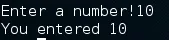

There we have it! We used try and except in order to detect and handle errors! Let's end it by wrapping it all up!
Wrapping It Up
We covered quite a few new concepts in this article, but I hope the messages got across well. We covered the concepts of errors not being all that bad, how to diagnose an error from the error message, and how to look for areas of failure within our code. We also made a small script incorporating try and except, our error handling duo. Let's get on to the exercise!
The Exercise
Alright, now that we know how to properly deal with errors, I would like you to make and handle as many errors as you can. Set yourself up for failure, then save yourself with try and except. As always, don't be afraid to experiment!
Feedback!
That's it for this one, I hope you'll come back next time for your python's next training session!
Thanks for reading!
-Defalt
Comments
Be the first, drop a comment!