Welcome back! Sorry for being so quiet, I've been rather busy with this project lately! Anyways, in the last iteration of how to train your python, we covered lists. Today we'll be introducing iteration and the two loops python has to offer, for and while. Also, we'll be covering a couple general use functions. So, let's get started!
Introduction to Iteration
You may be asking "What is iteration?". Well, iteration (in scripting terms) is when we loop through something, one element at a time. If you didn't read the last article about lists and indexing, I suggest you do so before continuing here, as this will make better sense with the concepts we covered there.
When we iterate through something, we simply loop through all of it's values and feed those into a set of instructions. We can do whatever we want with loops, they're just a way for us to isolate each element without having to call them out individually. Loops are one of those things that we'll use throughout our ENTIRE scripting career. Before we get into loops, we'll have to cover some functions that we often use along with them, so let's do that now.
General Functions
Let's start by asking "What is a function?". Well, a function is something that returns a result. We'll be learning much more about them later, but all we have to know now is that a function is something we feed information into, and get information out of. Now let's cover the two function we'll be using rather frequently with loops...
- range()
- len()
Lets start with the range() function. This simply returns an exclusive list of numbers based on what we give it. It can take a maximum of three arguments or a minimum of one. We'll demonstrate my giving it two, and use it later with one. Let's make a list of numbers using range, shall we?

We gave the range() function the numbers 1 and 10 as arguments. The start (minimum) number is 1, and stop (maximum) number is 10. Since this is exclusive, it produced a range of numbers 0 to 9. It's noting that that if one argument is given to range(), it will use it as the stop number, and set the start number to 0. Now let's go on to the len() function...
For demonstrating the len() function, and for use through the rest of this article, we'll be setting our test variable to a list of the numbers 1, 2, and 3...

The len() function is extremely simple, it takes one argument, a variable name. len() simply returns the length of the given variable, whether it be a string, list, or dictionary (which we'll cover later). Let's use it so we can see the result...

We see here that the result is right, the number returned to us matches the number of elements in the list.
Now that we've covered the two individually, let's put them together and see what happens...

This result may not seem important, but if you remember from the last article, these are the index numbers for our test list. This is rather useful as it allows us to use object oriented methods on these elements. Now that we know the basic functions we need, let's move on to our first loop, for.
The For Loop
The for loop. What makes for so special is that it lets us call out list elements one at a time. Also, a for loop only goes iterates through everything once. This means it will stop once it reaches the end of our list. Let's use the for loop, then break it down and explain the syntax...

Now, we start by entering for, which will designate that we wish to use a for loop, then we enter our element variable name. Usually we use "i", this is simply the variable name that the element will temporarily take, as you can see inside of the loop when we print i, we're printing the element that currently occupies that name. After we specify the name, we tell it what we would list to iterate through, in this case our test list. Then we enter a colon. Below that and one tab in, we enter what we want to to with each element we iterate through, here, we're just printing it.
Now let's use our functions for earlier and see what we can do with them...
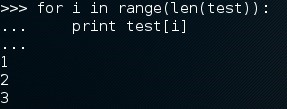
We've use our range() and len() functions to make a list of the index numbers of our test list. Instead of iterating through the test list itself, we're iterating through this list, and calling the elements using the index numbers under the name i. In this example, the result is the same, but this allows us much more freedom to manipulate lists using this loop.
Now that we've covered for, let's go on to the other loop we can use... while.
The While Loop
The while loop is a bit more unstable and trickier to use. The for loop is meant to go through something, the while loop is not. There is no variable name, or list to give it. While loops take conditional statements. Just like if we're making an if statement.
While will only stop under two circumstances, it's conditional statement becomes false, or it is manually stopped. If the conditional statement always remains true, it will go on forever. Let's reset our test variable to just equal True. Now let's build our while loop...

When we execute this, it will print "Test is equal to True!" until we manually stop it. Usually we would use while for things like taking user input, if input need to be given continuously. Let's execute this loop and see what happens...

This went on for much, much longer. But I could only fit this much into the screen-shot. While loops can be volatile if used incorrectly, but they can also make for some nasty bomb-type scripts. That does it for the content portion of this article, so let's get to the exercise!
The Exercise
Use both loops to do something! It doesn't matter what it is, just prove that you understand that concept of loops and iteration! Remember, don't be afraid to experiment!
Feedback!
Sorry about being to quiet, I'll be post more frequently now that I've gotten over some bumps in this project. So, expect more training soon, and I'll see your for your python's next training session!
Thank you for reading!
-Defalt
Just updated your iPhone? You'll find new emoji, enhanced security, podcast transcripts, Apple Cash virtual numbers, and other useful features. There are even new additions hidden within Safari. Find out what's new and changed on your iPhone with the iOS 17.4 update.
10 Comments
Got a little confused with the range(len(variable) function at first, but as I understand it, the function returns our list indexed? Whether our list is a list of strings or integers doesn't matter right?
Correct.
I'm having trouble with the while loop. It's not working for me
>>> while test==True:
... print "Test is equal to True!"
...
>>>
It only does that^
Have you set the test variable and check if is it true first?
Try this:
>>> test = 1
>>> test >= 1
True
>>> while test==True:
... print " test is true"
It works like a charm.
Same is happening with me... the loop While is like
>>> while test == True:
... print "The Test is Awesomely True"
...
>>>
The_Unknown
You have to do like mr dorian. First you have to define test = True and then you can execute the while function
I did what mr Dorian did and it still all it did was put more >>> on every line.
I'm using an app on my phone that uses 2.5.6
>>> test = 1,2,3
>>> test >= 1,2,3
True
While test ==True:
... print "test is true"
...
>>>
>>>
It just keep doing the >>> at the end.
Never mind. I got it to work.
well bro there is a problem that i don't understand fully yet but
python doesn't take test = 1,2,3 you have to properly tell this either make test
test = 1 or test= True
test = 0 or test = False
#!/usr/bin/python
lisst = "Apple","PineApple","Sweet","light","Orange","Wine"
while True:
print"""
(1)Insert
(2)Append
(3)Remove
(4)show list
"""
uinp = int(rawinput("UserInput: "))
if u_inp == 1:
lisst.insert(int(rawinput("place : ")),rawinput("Name : "))
for i in range(len(lisst)):
print (i, lissti )
elif u_inp == 2:
lisst.append(raw_input("Name : "))
for i in range(len(lisst)):
print (i, lissti )
elif u_inp == 3:
lisst.remove(raw_input("Name : "))
for i in range(len(lisst)):
print (i, lissti )
elif u_inp == 4:
for i in range(len(lisst)):
print (i, lissti )
else:
print("Wrong Opt")
i used the same script that i made on if statement and changed it i didn't wanted to remove if statement to make your effort of writing this worth it so i tried everything i could think of and made it and still trying to make it more worthy ...
Share Your Thoughts