Welcome back! In the last training session, we covered logical and membership operators. We're going to switch gears and expand our knowledge of lists.
More specifically, we're going to be discussing list slicing and comprehension, in that order. You may be asking what these are, so let's get some background before we get into the code.
What Is List Slicing?
Well, let's think of the definition of the word slice; to cut something into smaller slices. When we use list slicing, we're cutting a list based on what we want (Such as where to start, stop, and what increment to slice by). List slicing can be useful in some cases, but I don't really use it that often. None-the-less, this is one of the more advanced list aspects, so we need to know it!
What Is List Comprehension?
List comprehension is generating a list based on an existing list. We'll be doing two examples here. One example for numbers, and one example for strings. One thing that needs to be understood about list comprehension is that a newly generated list must be generated based on a preexisting list. We'll see this displayed in our examples
Now that we've discussed some base knowledge of our two advanced list aspects, let's get into the code!
A Side Note
Lists can be indexed backwards, starting at -1 (Last element) and increasing (technically decreasing) by -1. So -1 would be the last element, -2 would be the second to last element. This can be very useful.
List Slicing
Let's jump into list slicing!
If we're going to slice a list, we'll need a list to slice! Let's go ahead and make a list. For the sake of demonstration, we'll be writing two sentences, one word at a time, in a single list. We'll then slice the list to isolate the two sentences. Let's go ahead and make our list:

Alright, now that we have our list, let's slice out our first sentence. Let's take a look at the code, and then discuss what happened. So, let's slice out our first sentence:

In the demonstration above, we've assigned our first slice to a new variable named "sentence1", then we printed the value of this new list. Let's take a moment to discuss the syntax for list slicing.
When slicing a list, we must call our list, in this case it was "test", followed by our requirements for slicing. These must be encased in brackets []. There are a few arguments that we can/must give, they are (in order):
- Index to start slicing, this goes in the first place of our slice.
- Index to stop slicing, this goes in the second place of our slice.
- Finally, we have the increment to slice by, this one is optional. (This increment is known as the "step"
So, in the end, it should look like this:
LISTNAME[START:STOP:STEP]
Alright, let's apply this knowledge and quickly slice out our second sentence. After we've sliced it, we'll print both our new lists to see our isolated sentences:
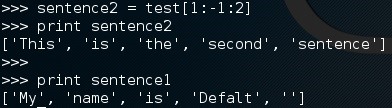
There we go! We've got list slicing down pat! Let's move on to list comprehension!
List Comprehension
Simply put, list comprehension is generating a new list based on an old one. We'll be generating two lists today, one with numbers, and one with strings. Let's get started with making our first list out of numbers. We'll be using the range() function in order to generate a temporary list of numbers for our list comprehension, so let's make our list:
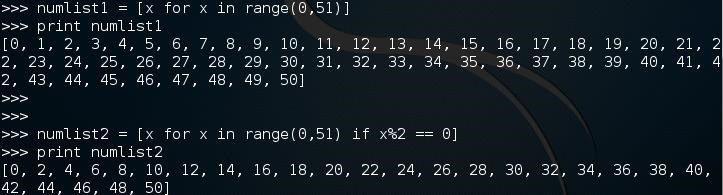
Let's break down and review this syntax so we know what's happening. We've given our temporary variable name "x" followed by a for loop that iterates through a range of 0 to 51. This is encased in brackets, and when we executed it, it generated a list of numbers 0 to 50. Then we made a second list, and we added a filter. This filter was "if x%2 == 0". What this means is that we only want x if it's even divisible by two. In other words, we only want even numbers using this filter.
The way the filters work is that if the outcome of the given conditional statement is true, then x will be included in the resulting list. Let's move on to making our next list with strings.
Let's make a list of strings with random capitalization:

Alright, now that we have our list. Let's use list comprehension to generate a list of all the same strings, but with every string in lowercase:

Alright! What we've done here is very similar to the numbers example, but we've demonstrated that our temporary variable can be treated like anything!
Let's wrap all this up, shall we?
Wrapping It Up
We've successfully expanded our knowledge of lists to the more advanced topics! This is a big step in learning how to use lists! The things we covered here today may seem like they may not be used all that often, but with a little creativity we should be able to put them to good use!
Feedback!
Let me know what you think! If you have any questions feel free to leave them here or to message me about them! I'll be happy to help you with anything you need!
I'll see you for your python's next training session!
Thank you for reading!
-Defalt
Just updated your iPhone? You'll find new emoji, enhanced security, podcast transcripts, Apple Cash virtual numbers, and other useful features. There are even new additions hidden within Safari. Find out what's new and changed on your iPhone with the iOS 17.4 update.
1 Comment
These tutorials are amazing, thank you so much!
Share Your Thoughts