Welcome back, my greenhorn hackers!
I recently began a series on scripting and have received such positive feedback that I'm going to keep this series going. As I've said before, to graduate from the script kiddie to the pro hacker, you'll need to have some scripting skills. The better you are at scripting, the more advanced your hacking. Ultimately, we are leading up to developing the skills to build your own zero day exploits.
I left off teaching you the basics of shell scripting in BASH and built a simple script that utilized nmap with our user-supplied inputs to scan for open ports on a range of IP addresses. I hope you saved that script, as we'll be building upon it here, adding additional functionality.
Step 1: Open a Text Editor
The first step in building any script is to open a text editor. You can use any text editor, but I will be using kwrite in the KDE version of BackTrack (if you are using the GNOME version, gedit works just as well).
Now, in this lesson, we'll be studying conditional statements. Those are statements within our script that enable us to make decisions. In other words, "If this happens, do this. If the other happens, then do that."
Step 2: If...then...else
The most basic type of conditional statement that is available in nearly every programming language is the if...then...else. This enables us to check for a condition (if) and if it is true, then execute some statement or statements, or else do something different. Its basic form is:
- if <a conditional statement that evaluates to true or false>
- then <statements to execute when true>
- else <statements to execute if false>
- fi
The else is optional, as the conditional statement will run without the else clause. In BASH shell scripting, every if..then must be closed with a "fi" or the reverse of the "if."
Step 3: Let's Add a Conditional to Our Scanner Script
Now that we have the basic concept of a conditional script, let's add one to our scanning script named Scannerscript. Let's ask the user whether they want to use nmap or hping3 to scan the target. If they say they want nmap, then we can execute the original part of our script that does the nmap scan. If they want to use hping3, we will have to add a new section to gather info and then run an hping scan. So, our script structure should look like this:
- if you want to run nmap
- then run nmap prompts and commands
- else run hping prompts and commands
Step 4: Create Our Conditional Statement
Now, let's edit our Scannerscript to give the user a choice of either using nmap or hping to scan. First, we need ask the user which they want to use with an echo statement.
- echo "Would you like to scan using nmap or hping"
This simply asks the user to enter which scanner they would like to use and enter it from the keyboard. Next, we need to capture their input into a variable named scanner.
- read scanner
Now, comes the key part of our conditional statement, the if...then...else. We create a statement that checks to see what value the user entered when prompted, and then sends our script to either the nmap section, or our yet to be created hping section. We can do this with the following statement:
- if "$scanner" = "nmap" then

Please note a few things here.
First, the if is lowercase. Anything else will throw a "command not found" error. Second, I used double quotation marks around the value of the variable scanner. This is to indicate that I want a string to compare to the string "nmap". Third, when retrieving the value of a variable, I precede it with a $ sign.
Step 5: Build the Hping Scan Section
Now that we've developed our basic structure and logic of our conditional statement, let's build the hping section that will be executed if the user chooses they want to run an hping scan. Let's start by prompting the user for which IP address they want to scan and capturing the data into a variable called IPaddress.
- echo "Which IP address would you like to scan? :
- read IPaddress
Next, let's prompt the user which port they would like to scan and capture the data into a variable called hpingport.
- echo "What port would you like to scan for ?:
- read hpingport
Finally, let's ask the user how many packets they would like to send. Remember, hping continues to send packets until stopped just like the ping command in Linux (and unlike the ping command in Windows that only sends 4 packets and then stops). We can determine how many packets to scan by using the -c switch in the hping command. So, let's ask the user how many packets they would like to send and gather that info into a variable called packets.
- echo "How many packets would you like to send?"
- read packets
Step 6: Create Our hping3 Scan Command
Now we have all the information we need from the user and saved it into variables to create our hping3 command.
- hping3 -c $packets $IPaddress -p $hpingport > hpingscan

This statement says run the hping3 command and send $packets number of packets to the $IPaddress IP address, scanning for $hpingport port open and send it all to a file named hpingscan.
Step 7: Let's Test It
To run this script and see whether it works, let's first re-save it as Scannerscript. Now let's open a terminal and run it by typing;
- ./Scannerscript
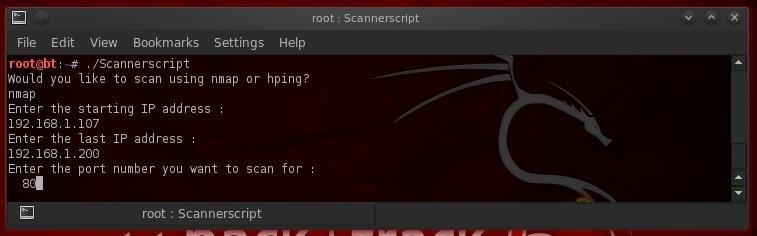
As you can see, when I asked it to run the nmap scan, it did just that.
Now, comes the critical part. Let's ask our Scannerscript to run an hping scan. To do so, it will have to check to see whether the user requested a nmap or hping scan, and if an hping scan, skip over the nmap section of our code and go directly to the hping section. This utilizes our conditional statement. Let's give it a try.
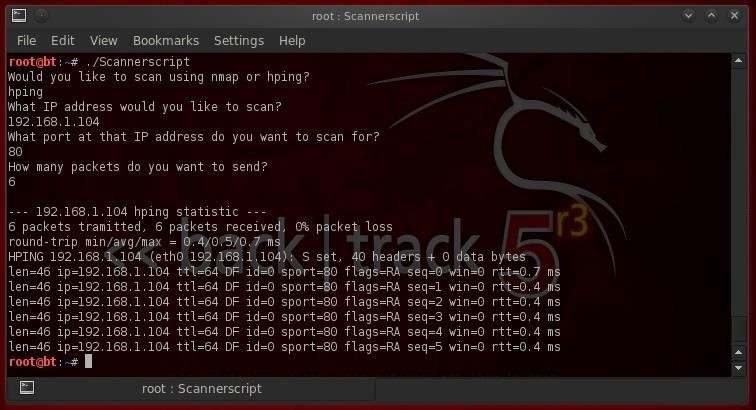
Success! Our script enabled the user to select either a nmap or a hping scan by using the conditional if...then...else!
In future tutorials, we will continue to build functionality to this script, so make sure you save it. We will eventually look at other scripting languages such as Perl, Ruby, and Python so we can build our own zero day exploit!
Just updated your iPhone? You'll find new emoji, enhanced security, podcast transcripts, Apple Cash virtual numbers, and other useful features. There are even new additions hidden within Safari. Find out what's new and changed on your iPhone with the iOS 17.4 update.
10 Comments
Thanks for yet another great tutorial! I really enjoy reading your articles as they always contain lots of useful information.
Is it on purpose that the textual example, different from the screenshot, in Step 4, is without the brackets and semi-colon?
Could be the formatting of the text editor on this site. The site lacks a code format for us folks. I get some odd results when I post code as well. Like the fork bomb I had posted in my profile bio( ;-p) was wrong after refresh.
Andreas:
Yes, the editor for this website often removes special characters, but the screenshot IS correct.
OTW
Amazing! thanks for the great tutorial, i'll be sure to check in for more tutorials!
hello sir OTW,
I'm wondering, how can i make the script execute other commands in different terminal ? (like instantly open a new terminal and execute the command there)
For example, the 1st terminal is where the prompt is, then the 2nd terminal is executing airodump-ng, then the 3rd one is executing aireplay-ng...
You have to call a new terminal in your script and give it a command. There are a couple of different ways you could do this depending on what your setup is.
gnome-terminal -e <enter stuff here>
knosole -e <enter stuff here>
xterm -e <enter stuff here>
terminator -e <enter stuff here>
Only problem is most of them will close right after your command completes. I normally get around this with something like:
gnome-terminal --window -x bash -c "nmap -sT 192.168.1.1; bash"
Of course replace the nmap command with what ever you need. Unless someone else has a better way of doing it.
hello friends,
i get some trouble to create the explained script with diff. error msg. like:
failed to resolve "="...
and if change the values i get trouble with "else"
pls, make the script clear
#! /bin/bash
echo "Would you like to scan using nmap or hping?"
read scanner
if ( "$scanner" = "nmap" );then
echo "Enter the starting IP address :"
read FirstIP
echo "Enter the last IP address :"
read LastIP
echo "Enter the port number you want to scan for :"
read port
nmap -sT $FirstIP-$LastIP -p $port -oG web
cat web | grep open >web1
cat web1 | cut -f2 -d":" | cut -f1 -d"(" >web2
cat web2
else
echo "What IP address would you like to scan?"
read IPaddress
echo "What port you want to scan for?"
read hpingport
echo "How many packets you want to send?"
read packets
hping3 -c $packets -S $IPaddress -p $hpingport>hpingscan
cat hpingscan
fi
thank you so much
It would help us diagnose your problem is you gave us a screenshot.
here's the screenshot
Problem solved :)
Hi!
I created a script using your guidelines but after running it I always get an error like: line 22 "unexpected syntax error near else". If I add ":" after that "else" it then shows the exact error but for the next "fi" so I need to add another ":" and then again for the next "else". Also I can t end the script because of the last fi... adding ":" gives "Unexpected end of file".
Here is the script:
#! /bin/bash
echo "+------------+"
echo "| HELLO MASTER |"
echo "+------------+"
sleep 1
echo "Please press 1 for Nmap scan, or 2 for hping scan"
read choice
if "$choice" = "1" : then
echo "Enter the starting IP address: "
read startingip
echo "Enter the last IP address: "
read lastip
echo "Do you want to perform an OS scan?"
read OS
if "$OS" = "yes" : then
echo "Starting..."
sleep 1
nmap -O $startingip- $lastip -oG scan
cat scan | grep open > scan1
cat scan1 | cut -f2 -d":" | cut -f1 -d" (" > scan2
cat scan2
else
echo "Starting..."
nmap $startingip- $lastip -oG scan
cat scan | grep open > scan1
cat scan1 | cut -f2 -d":" | cut -f1 -d" (" > scan2
cat scan2
fi
else
echo "What IP address would you like to scan?"
read IPaddres
echo "What port would you like to scan?"
read port
echo "How many packets do you want to send"
read packets
hping3 -C $packets -S $IPaddres -p $port > hpingscan
cat hping scan
fi
Please help me! :)
Share Your Thoughts