Welcome to another C tutorial. We will be looking at arrays, discussing what they are, when they are used and their special relationship with the char variable. Let's begin.
What Is an Array?
Think of an array as a sort of container or like... a buffer. They allow us to be able to store and hold items inside them like ice trays can hold water or ice. In fact, representations of arrays are sort of like an ice tray with boxes which hold values. They are great when you want to group the same type of data together to store information. Let's see it drawn out.
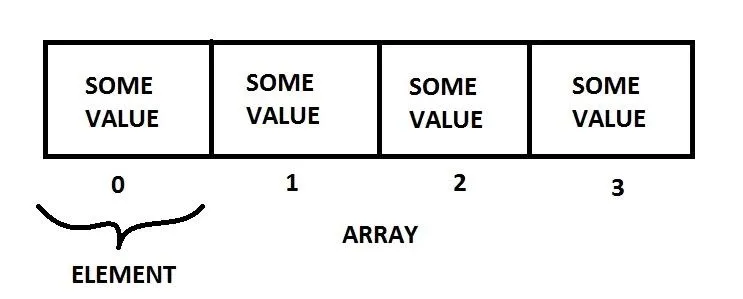
As you can see, arrays also start at 0! It has 4 "elements" from 0 to 3, each holding some value. If you're not used to this "starting at 0" notation, you'd better start now.
But why on earth would we need arrays? We already have variables which can hold values, what's the point of this? Well, look at how convenient it is, how nicely arranged and organized it is. Wouldn't you rather have this than 4 entirely different variables which don't have any proper relation to each other? With arrays, each element has its own "index" with which you can reference specific elements. Let's have a look at how we can write it up in code.
Example Code
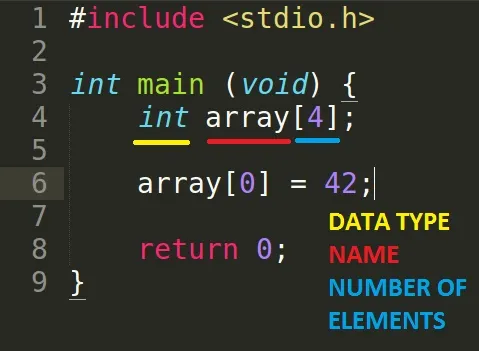
To declare an array, you must first specify what data type the array will hold and how much space you require. Once you declare the size of the array, you will not be able to add any more. Here, I have declared an array to hold 4 ints, i.e. starting from element 0 to 3. How big is the array itself? A graphical representation can be seen below.
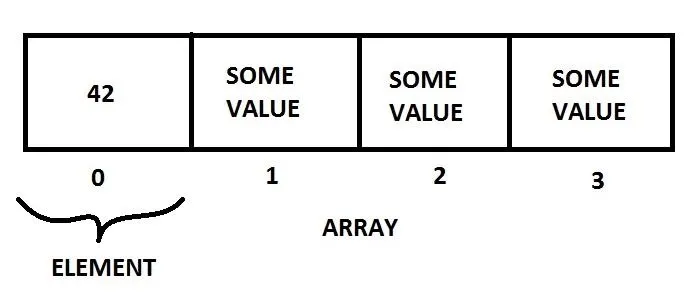
When assigning values to an element, you must refer to the array and the corresponding index. I have assigned element 0 to hold the value 42 by typing the name of the array and the element number followed by the value.
But hang on, I didn't set any values to the other elements. What values do they hold? 0? Well, we can't really be sure (I'll explain this when we get to memory). It depends if your compiler will set it to 0 for you or not. Can you rely on the fact that your compiler will zero the uninitialized elements? No. Should you? No. Never. You must explicitly zero it out yourself to be sure.
Character Arrays
Character arrays are pretty much the same thing as an integer array. The only difference is the content they hold... and one other thing. The char data type is special with arrays because in C, char arrays are what make up strings. Let's see an example.
Example Code
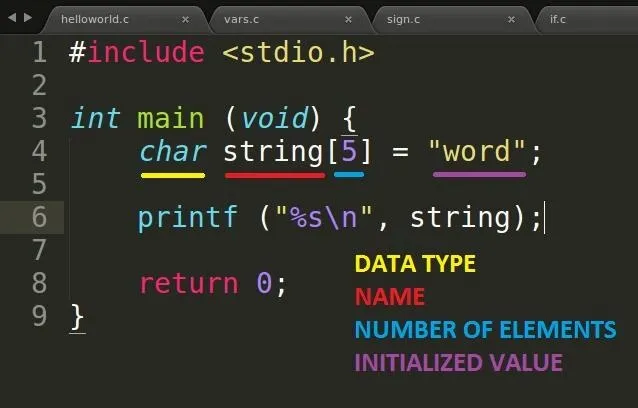
The example code shows a character array with 5 elements initialized with the value "word". Why did I initialize string with 5 elements when "word" has only 4 characters? Okay, so, listen up carefully and pay attention. The reason why I have the extra element there is because what identifies a string as a string, instead of just random characters in an ordered fashion, is something called a null terminator which signifies the termination or the end of a string. The printf with the %s format specifier (string) will print out the characters in the array until it reaches the null terminator which is when it knows that it should stop. What happens when there is no null terminator? Well, take a wild guess! If you're starting to piece the puzzle together, just be a bit more patient! If you're not, well, you'll know soon enough.
Note: It is not possible for you to assign a string to a character array without initializing it in the same line as the declaration. There is a reason for this and I will explain it soon enough.
Conclusion
Sorry for ending this on a cliff hanger, for those who know what's up. Play around with strings for a bit, try and experiment crazy things like leaving out the null terminator and then printing it. It might break something or not. Who knows? Until next time!
dtm.
Comments
Be the first, drop a comment!